首页c语言计算返回链栈中的结点个数代码
c语言计算返回链栈中的结点个数代码
时间: 2024-10-22 18:16:18 浏览: 25
在 C 语言中,计算链栈(通常指单向链表实现的栈)中的节点个数,可以编写一个递归或非递归的函数。这里是一个简单的非递归版本的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 函数用于计算链栈的节点数
int count_nodes(Node* top) {
if (top == NULL) { // 判断链表是否为空
return 0; // 空链表,返回0
} else {
return 1 + count_nodes(top->next); // 非空链表,返回当前节点加上剩余节点数
}
}
int main() {
// 初始化链栈或其他方式填充节点
Node* stack = create_stack(); // 假设create_stack()创建了链栈
// 计算节点数并打印结果
int node_count = count_nodes(stack);
printf("链栈中有 %d 个节点。\n", node_count);
// 清理链表(如果有需要)
destroy_stack(stack);
return 0;
}
```
在这个例子中,`count_nodes`函数通过遍历链表的每个节点并递归地增加计数来计算总数。如果链表为空(`top`为`NULL`),则停止递归并返回0。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
大家在看
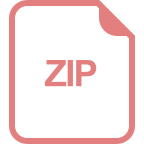
GL3231S USB4.0读卡器Layout和原理图及相关的FW
GL3231S USB4.0读卡器Layout和原理图及相关的FW
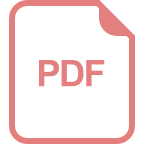
keb变频器 f5中文说明书-维修安装调试
本说明书详细介绍了keb变频器 f5 相关参数,使用方法和异常调试,如果想要更多变频器技术说明书、请访问CSDN下载频道
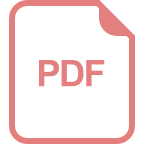
IPC-7351 使用说明
IPC-7351 软件,零件封装库制作标准软件的中文使用说明。
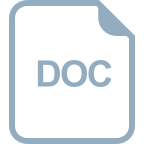
实验二DML语言一(数据插入、修改和删除.doc
大学在校生以及从事互联网开发学习人员
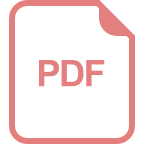
ZYNQ_7020核心板原理图.pdf
XC7Z020处理器,外扩DDR3 SDRAM,Winbond flash,eMMC,PHY等设计资源
最新推荐

C语言计算代码执行所耗CPU时钟周期
这个计数器的值是递增的,因此可以通过比较开始和结束时刻的值来计算代码执行的时钟周期数。 代码如下: ```c #include #include LARGE_INTEGER _start = { 0 }, _end = { 0 }; void start_timer() { __asm{ ...

C语言中使用lex统计文本文件字符数
在C语言中,使用lex工具进行文本文件字符数统计是一种常见的词法分析应用。Lex是一个词法分析器生成器,它可以读取一个包含模式规则的输入文件(通常以`.l`为扩展名),并生成相应的C代码,这个C代码能够识别输入流...

C语言统计一篇英文短文中单词的个数实例代码
C语言统计一篇英文短文中单词的个数实例代码 本文详细介绍了使用C语言统计一篇英文短文中单词的个数的实例代码,代码简单易懂,具有参考借鉴价值。下面我们将对代码进行详细的解释和分析。 首先,我们需要了解统计...

C语言中计算二叉树的宽度的两种方式
在C语言中,计算二叉树的宽度是一个常见的问题,主要涉及到数据结构和算法的知识。二叉树是一种每个节点最多有两个子节点的数据结构,通常分为左子节点和右子节点。计算二叉树的宽度,即找出树中最宽的一层包含的...

C语言实现将字符串转换为数字的方法
在C语言中,将字符串转换为数字是一项常见的任务,这对于处理用户输入或解析文本数据至关重要。本文主要讨论了如何利用C语言的标准库函数将字符串转换为整数、长整数和浮点数。 首先,我们关注`atoi()`函数,它是...
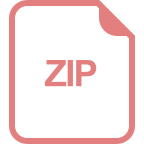
免安装JDK 1.8.0_241:即刻配置环境运行
资源摘要信息:"JDK 1.8.0_241 是Java开发工具包(Java Development Kit)的版本号,代表了Java软件开发环境的一个特定发布。它由甲骨文公司(Oracle Corporation)维护,是Java SE(Java Platform, Standard Edition)的一部分,主要用于开发和部署桌面、服务器以及嵌入式环境中的Java应用程序。本版本是JDK 1.8的更新版本,其中的241代表在该版本系列中的具体更新编号。此版本附带了Java源码,方便开发者查看和学习Java内部实现机制。由于是免安装版本,因此不需要复杂的安装过程,解压缩即可使用。用户配置好环境变量之后,即可以开始运行和开发Java程序。"
知识点详细说明:
1. JDK(Java Development Kit):JDK是进行Java编程和开发时所必需的一组工具集合。它包含了Java运行时环境(JRE)、编译器(javac)、调试器以及其他工具,如Java文档生成器(javadoc)和打包工具(jar)。JDK允许开发者创建Java应用程序、小程序以及可以部署在任何平台上的Java组件。
2. Java SE(Java Platform, Standard Edition):Java SE是Java平台的标准版本,它定义了Java编程语言的核心功能和库。Java SE是构建Java EE(企业版)和Java ME(微型版)的基础。Java SE提供了多种Java类库和API,包括集合框架、Java虚拟机(JVM)、网络编程、多线程、IO、数据库连接(JDBC)等。
3. 免安装版:通常情况下,JDK需要进行安装才能使用。但免安装版JDK仅需要解压缩到磁盘上的某个目录,不需要进行安装程序中的任何步骤。用户只需要配置好环境变量(主要是PATH、JAVA_HOME等),就可以直接使用命令行工具来运行Java程序或编译代码。
4. 源码:在软件开发领域,源码指的是程序的原始代码,它是由程序员编写的可读文本,通常是高级编程语言如Java、C++等的代码。本压缩包附带的源码允许开发者阅读和研究Java类库是如何实现的,有助于深入理解Java语言的内部工作原理。源码对于学习、调试和扩展Java平台是非常有价值的资源。
5. 环境变量配置:环境变量是操作系统中用于控制程序执行环境的参数。在JDK中,常见的环境变量包括JAVA_HOME和PATH。JAVA_HOME是JDK安装目录的路径,配置此变量可以让操作系统识别到JDK的位置。PATH变量则用于指定系统命令查找的路径,将JDK的bin目录添加到PATH后,就可以在命令行中的任何目录下执行JDK中的命令,如javac和java。
在实际开发中,了解并正确配置JDK对于Java开发者来说是一个基础且重要的环节。掌握如何安装和配置JDK,以及如何理解JDK中的源码和各种工具,对于进行Java编程和解决问题至关重要。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire

【提升效率与稳定性】:深入掌握单相整流器的控制策略
# 摘要
单相整流器作为电力电子技术的基础设备,广泛应用于电力系统和工业自动化领域。本文首先概述了单相整流器的基本概念,详细分析了其工作原理,包括电力电子器件的角色、数学模型以及控制策略。随后,针对控制技术进行了深入探讨,包括传统控制技术如PID控制和高级控制策略如模糊逻辑控制及自适应控制的应用。进一步地,本文提出了一系列提升单相整流器性能的方法,如效率提升策略、系统稳定性措施,以及性能评估与优化方案。最后,文章探讨了单相整流器在不同领域的应用案例,并对新兴技术与市场趋势进行了展望。
# 关键字
单相整流器;电力电子器件;数学模型;控制策略;性能提升;应用案例
参考资源链接:[单相PWM整

你看这是ashx映射的cs文件初始代码,你看这里边根本就没有写对action参数进行任何操作但你.ashx?action=submit这样去做他就能返回出数据这是为什么
在ASP.NET中,.ashx文件(也称为HTTP处理程序)用于处理HTTP请求并返回响应。即使在初始代码中没有对action参数进行任何操作,系统仍然可以通过默认的ProcessRequest方法处理请求并返回数据。
当你在URL中传递参数(如?action=submit)时,这些参数会被包含在请求的查询字符串中。虽然你的代码没有显式地处理这些参数,但默认的ProcessRequest方法会接收这些参数并执行一些默认操作。
以下是一个简单的.ashx文件示例:
```csharp
<%@ WebHandler Language="C#" Class="MyHandler" %>
us
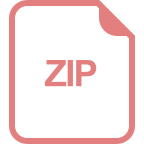
机器学习预测葡萄酒评分:二值化品尝笔记的应用
资源摘要信息:"wine_reviewer:使用机器学习基于二值化的品尝笔记来预测葡萄酒评论分数"
在当今这个信息爆炸的时代,机器学习技术已经被广泛地应用于各个领域,其中包括食品和饮料行业的质量评估。在本案例中,将探讨一个名为wine_reviewer的项目,该项目的目标是利用机器学习模型,基于二值化的品尝笔记数据来预测葡萄酒评论的分数。这个项目不仅对于葡萄酒爱好者具有极大的吸引力,同时也为数据分析和机器学习的研究人员提供了实践案例。
首先,要理解的关键词是“机器学习”。机器学习是人工智能的一个分支,它让计算机系统能够通过经验自动地改进性能,而无需人类进行明确的编程。在葡萄酒评分预测的场景中,机器学习算法将从大量的葡萄酒品尝笔记数据中学习,发现笔记与葡萄酒最终评分之间的相关性,并利用这种相关性对新的品尝笔记进行评分预测。
接下来是“二值化”处理。在机器学习中,数据预处理是一个重要的步骤,它直接影响模型的性能。二值化是指将数值型数据转换为二进制形式(0和1)的过程,这通常用于简化模型的计算复杂度,或者是数据分类问题中的一种技术。在葡萄酒品尝笔记的上下文中,二值化可能涉及将每种口感、香气和外观等属性的存在与否标记为1(存在)或0(不存在)。这种方法有利于将文本数据转换为机器学习模型可以处理的格式。
葡萄酒评论分数是葡萄酒评估的量化指标,通常由品酒师根据酒的品质、口感、香气、外观等进行评分。在这个项目中,葡萄酒的品尝笔记将被用作特征,而品酒师给出的分数则是目标变量,模型的任务是找出两者之间的关系,并对新的品尝笔记进行分数预测。
在机器学习中,通常会使用多种算法来构建预测模型,如线性回归、决策树、随机森林、梯度提升机等。在wine_reviewer项目中,可能会尝试多种算法,并通过交叉验证等技术来评估模型的性能,最终选择最适合这个任务的模型。
对于这个项目来说,数据集的质量和特征工程将直接影响模型的准确性和可靠性。在准备数据时,可能需要进行数据清洗、缺失值处理、文本规范化、特征选择等步骤。数据集中的标签(目标变量)即为葡萄酒的评分,而特征则来自于品酒师的品尝笔记。
项目还提到了“kaggle”和“R”,这两个都是数据分析和机器学习领域中常见的元素。Kaggle是一个全球性的数据科学竞赛平台,提供各种机器学习挑战和数据集,吸引了来自全球的数据科学家和机器学习专家。通过参与Kaggle竞赛,可以提升个人技能,并有机会接触到最新的机器学习技术和数据处理方法。R是一种用于统计计算和图形的编程语言和软件环境,它在统计分析、数据挖掘、机器学习等领域有广泛的应用。使用R语言可以帮助研究人员进行数据处理、统计分析和模型建立。
至于“压缩包子文件的文件名称列表”,这里可能存在误解或打字错误。通常,这类名称应该表示存储项目相关文件的压缩包,例如“wine_reviewer-master.zip”。这个压缩包可能包含了项目的源代码、数据集、文档和其它相关资源。在开始项目前,研究人员需要解压这个文件包,并且仔细阅读项目文档,以便了解项目的具体要求和数据格式。
总之,wine_reviewer项目是一个结合了机器学习、数据处理和葡萄酒品鉴的有趣尝试,它不仅展示了机器学习在实际生活中的应用潜力,也为研究者提供了丰富的学习资源和实践机会。通过这种跨领域的合作,可以为葡萄酒行业带来更客观、一致的评价标准,并帮助消费者做出更加明智的选择。