java计算租赁价的编程练习,涉及Java中多态数组的应用。通过创建父类MotoVehicle和两个子类Car、Bus,以及测试类Test,实现了计算2辆宝马、1辆别克商务舱和1辆34座金龙5天的总租金功能。
时间: 2024-09-30 17:07:01 浏览: 41
在Java中,我们可以利用多态来编写一个租赁价格计算器的练习。首先,我们创建一个表示车辆基础特性的父类`MotoVehicle`,包含一些通用属性如品牌和基本费用等。然后,为具体的车型比如轿车`Car`和公交车`Bus`创建子类,分别继承自`MotoVehicle`并提供特有的属性和计算租赁费用的方法。
```java
// 父类 MotoVehicle
public class MotoVehicle {
private String brand;
private double baseRent;
// 构造函数和getter/setter...
public MotoVehicle(String brand, double baseRent) {
this.brand = brand;
this.baseRent = baseRent;
}
// 计算每天的基本费用
public double dailyRent() {
return baseRent;
}
}
// 子类 Car 和 Bus
public class Car extends MotoVehicle {
// 特有的属性如座位数...
private int seats;
// 构造函数和可能的特定计算费用方法...
public Car(String brand, double baseRent, int seats) {
super(brand, baseRent);
this.seats = seats;
}
// 汽车的每日费用可能基于座位数
@Override
public double dailyRent() {
return baseRent * (1 + seats / 100); // 示例,假设每增加100个座位多一单位费用
}
}
public class Bus extends MotoVehicle {
// 具有特定属性...
private int capacity;
public Bus(String brand, double baseRent, int capacity) {
super(brand, baseRent);
this.capacity = capacity;
}
// 公交车的费用可能基于容量
@Override
public double dailyRent() {
return baseRent * (1 + capacity / 500); // 示例,假设每增加500人多一定费用
}
}
// 测试类 Test
public class Test {
public static void main(String[] args) {
MotoVehicle[] vehicles = new MotoVehicle[3];
vehicles[0] = new Car("BMW", 200, 4); // 宝马轿车
vehicles[1] = new Bus("Buick", 300, 34); // 别克商务舱
vehicles[2] = new Bus("Golden Dragon", 500, 34); // 34座金龙巴士
int days = 5; // 租期为5天
double totalRent = 0;
for (MotoVehicle vehicle : vehicles) {
totalRent += vehicle.dailyRent() * days;
}
System.out.println("总租金: " + totalRent);
}
}
```
在这个例子中,`Test`类通过多态数组处理不同类型的车辆,调用的是各自子类的`dailyRent()`方法,从而实现了按车型计算租金的功能。运行`main`方法会得到所有车辆五天的总租金。
阅读全文
相关推荐
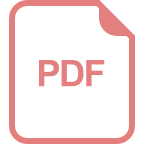
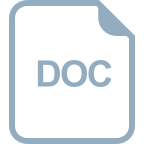


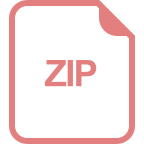
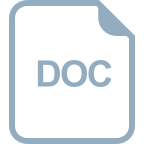
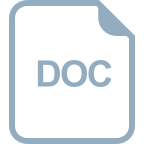
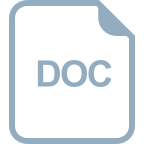
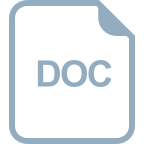
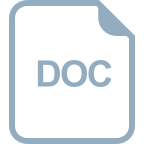
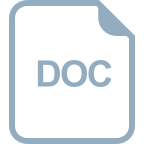
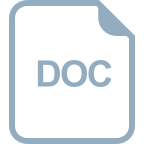
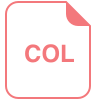
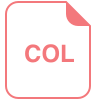
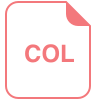



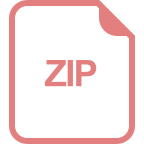