Python助学贷款的代码
时间: 2024-09-07 19:00:31 浏览: 55
编写一个简单的Python脚本来模拟助学贷款的过程可能包括以下几个步骤:
1. 定义类(如`Loan`)来表示贷款,包含属性如贷款金额、利率、还款期限等。
```python
class Loan:
def __init__(self, amount, interest_rate, term):
self.amount = amount
self.interest_rate = interest_rate
self.term = term
self.months = term * 12
def monthly_payment(self):
# 计算每月还款额
monthly_interest_rate = self.interest_rate / 1200
monthly_amount = (self.amount * monthly_interest_rate) / (1 - pow(1 + monthly_interest_rate, -self.months))
return monthly_amount
```
2. 可能还需要一个方法计算总利息:
```python
def total_interest(self):
return self.monthly_payment() * self.months - self.amount
```
3. 用户交互,获取贷款信息并显示结果:
```python
def main():
loan_amount = float(input("请输入贷款金额: "))
interest_rate = float(input("请输入年利率(例如5%): ")) / 100
term_in_years = int(input("请输入贷款期限(年): "))
loan = Loan(loan_amount, interest_rate, term_in_years)
print(f"每月还款额为: {loan.monthly_payment():.2f}")
print(f"总利息为: {loan.total_interest():.2f}")
if __name__ == "__main__":
main()
```
这个示例展示了如何基本地创建一个模拟助学贷款的模型。实际应用中,你可能需要添加更多的功能,比如贷款类型的选择(固定利率或浮动利率)、贷款审批条件检查等。
阅读全文
相关推荐
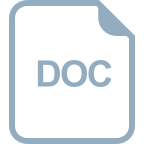
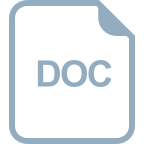
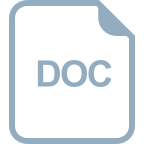


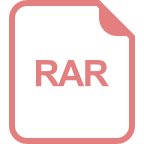
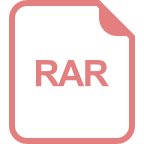
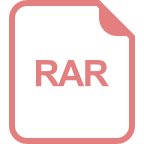
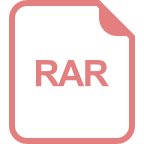
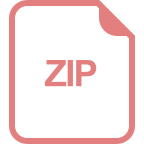
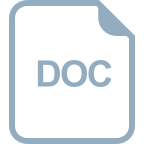
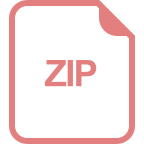
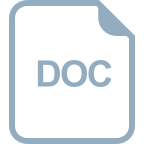
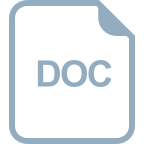
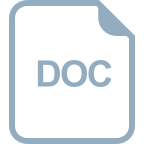
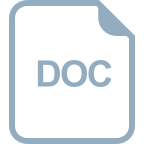
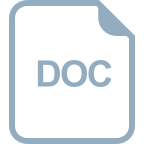
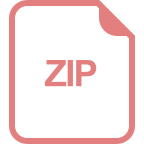