创建一个栈类,要求实现构造函数、判断栈是否为空、出栈、入栈等操作,通过数组来间接实现。使用using namespace std,写一个完整代码,要有析构函数和复制函数
时间: 2024-10-12 14:08:04 浏览: 21
创建一个基于数组的栈类,通常包含以下几个部分:
```cpp
#include <iostream>
using namespace std;
class Stack {
private:
int* arr; // 存储栈元素的数组
int top; // 栈顶指针
int capacity; // 栈的最大容量
public:
// 构造函数,初始化栈容量
Stack(int size) : arr(new int[size]), top(-1), capacity(size) {}
// 判断栈是否为空
bool isEmpty() const {
return (top == -1);
}
// 入栈操作
void push(int value) {
if (top < capacity - 1) {
top++;
arr[top] = value;
cout << "Pushed " << value << " to the stack." << endl;
} else {
cout << "Stack is full, cannot push." << endl;
}
}
// 出栈操作
int pop() {
if (!isEmpty()) {
int poppedValue = arr[top];
top--;
cout << "Popped " << poppedValue << " from the stack." << endl;
return poppedValue;
} else {
cout << "Stack is empty, nothing to pop." << endl;
return -1; // 返回一个特殊值表示空栈
}
}
// 复制构造函数
Stack(const Stack& other) {
if (other.isEmpty()) {
top = -1;
} else {
top = other.top;
arr = new int[capacity];
for (int i = 0; i <= top; i++) {
arr[i] = other.arr[i];
}
}
capacity = other.capacity;
}
~Stack() { // 析构函数,释放内存
delete[] arr;
}
// 复制赋值运算符
Stack& operator=(const Stack& other) {
if (this != &other) {
if (other.isEmpty()) {
top = -1;
} else {
top = other.top;
delete[] arr;
arr = new int[capacity];
for (int i = 0; i <= top; i++) {
arr[i] = other.arr[i];
}
}
capacity = other.capacity;
}
return *this;
}
};
int main() {
Stack s1(5); // 创建一个容量为5的栈
s1.push(1);
s1.push(2);
cout << "After pushing, stack contents: ";
while (!s1.isEmpty()) {
cout << s1.pop() << " ";
}
cout << endl;
Stack s2(s1); // 使用复制构造函数创建另一个栈并填充内容
return 0;
}
```
在这个例子中,我们实现了栈的基本功能,包括构造、判断空栈、入栈和出栈。同时,也提供了复制构造函数和赋值运算符。在`main()`函数里展示了栈的操作过程。
阅读全文
相关推荐
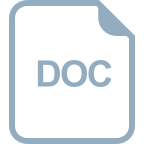
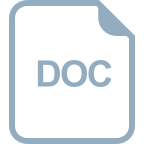
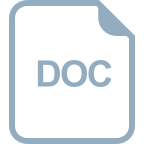


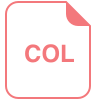
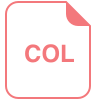
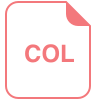
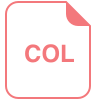
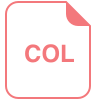
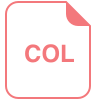
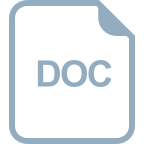
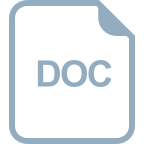
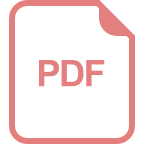
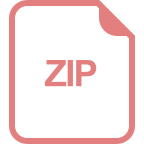
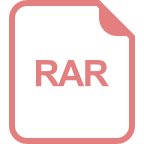
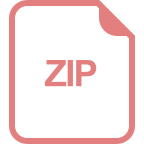
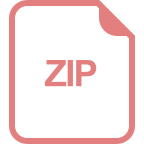
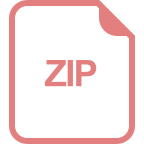