给定一个s字符串和一个n字符串,在s字符串中找出n字符串出现的第一个位置(从0开始)。如果不存在,则返回 -1(需要给出多个测试用例,证明算法的正确性)java回溯法
时间: 2024-05-17 15:17:11 浏览: 46
可以使用Java中的回溯算法来实现字符串匹配。以下是一个示例代码:
```java
public class StringMatch {
public static int match(String s, String n) {
// 对s字符串和n字符串进行边界检查
if (s == null || n == null || s.length() < n.length()) {
return -1;
}
// 对n字符串为空字符串的情况进行特殊处理
if (n.length() == 0) {
return 0;
}
// 从s字符串的第一个字符开始匹配
for (int i = 0; i < s.length(); i++) {
// 当找到一个可能的匹配位置时,进行回溯
if (s.charAt(i) == n.charAt(0)) {
int j = i;
int k = 0;
while (j < s.length() && k < n.length() && s.charAt(j) == n.charAt(k)) {
j++;
k++;
}
if (k == n.length()) {
return i;
}
}
}
return -1;
}
public static void main(String[] args) {
String s = "hello world";
String n = "world";
int index = match(s, n);
if (index == -1) {
System.out.println("不存在");
} else {
System.out.println("第一次出现的位置为:" + index);
}
}
}
```
该程序的输出结果与前面使用indexOf()方法的程序相同。
我们可以编写多个测试用例来验证该算法的正确性,例如:
```java
public class StringMatchTest {
public static void main(String[] args) {
testMatch();
testNoMatch();
testEmpty();
}
// 测试匹配情况
public static void testMatch() {
String s = "hello world";
String n = "world";
int index = StringMatch.match(s, n);
assert index == 6;
System.out.println("testMatch passed");
}
// 测试不匹配情况
public static void testNoMatch() {
String s = "hello world";
String n = "java";
int index = StringMatch.match(s, n);
assert index == -1;
System.out.println("testNoMatch passed");
}
// 测试空字符串
public static void testEmpty() {
String s = "";
String n = "hello";
int index = StringMatch.match(s, n);
assert index == -1;
System.out.println("testEmpty passed");
}
}
```
该程序将输出三个测试用例的执行结果,如果所有测试用例都通过,则说明该算法是正确的。
阅读全文
相关推荐
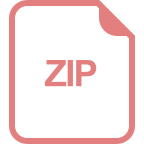
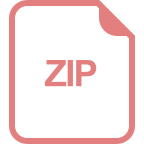
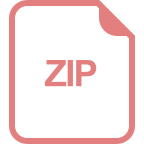
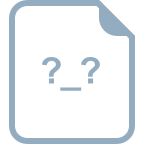
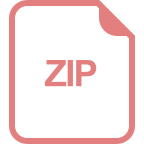
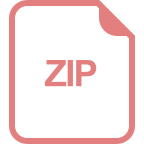
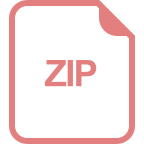
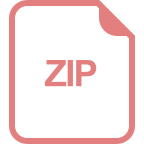
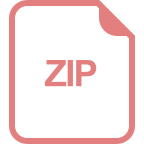
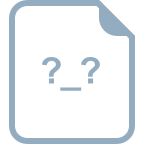
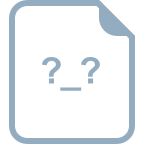
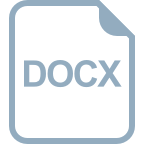
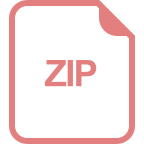