用c++实现顺序栈的基本操作并和括号匹配的问题且手动输入元素
时间: 2024-03-20 12:43:53 浏览: 70
好的,我来帮你实现。顺序栈是一种基于数组实现的栈结构,下面是它的基本操作:
1. 初始化栈
2. 判断栈是否为空
3. 判断栈是否已满
4. 入栈
5. 出栈
6. 获取栈顶元素
现在我们来实现一下这些操作:
```c++
#include<iostream>
using namespace std;
const int MAXSIZE = 100; // 栈的最大容量
// 栈结构体
struct Stack {
char data[MAXSIZE]; // 存放元素的数组
int top; // 栈顶指针,初始值为-1
};
// 初始化栈
void InitStack(Stack &S) {
S.top = -1;
}
// 判断栈是否为空
bool IsEmpty(Stack S) {
return S.top == -1;
}
// 判断栈是否已满
bool IsFull(Stack S) {
return S.top == MAXSIZE - 1;
}
// 入栈
bool Push(Stack &S, char e) {
if (IsFull(S)) {
return false; // 栈已满,无法入栈
}
S.data[++S.top] = e;
return true;
}
// 出栈
bool Pop(Stack &S, char &e) {
if (IsEmpty(S)) {
return false; // 栈为空,无法出栈
}
e = S.data[S.top--];
return true;
}
// 获取栈顶元素
bool GetTop(Stack S, char &e) {
if (IsEmpty(S)) {
return false; // 栈为空,无栈顶元素
}
e = S.data[S.top];
return true;
}
// 判断括号是否匹配
bool Match(char str[]) {
Stack S;
InitStack(S);
int i = 0;
while (str[i] != '\0') {
if (str[i] == '(' || str[i] == '[' || str[i] == '{') {
Push(S, str[i]); // 左括号入栈
} else if (str[i] == ')' || str[i] == ']' || str[i] == '}') {
char ch;
if (Pop(S, ch)) { // 右括号出栈
if ((ch == '(' && str[i] == ')') || (ch == '[' && str[i] == ']') || (ch == '{' && str[i] == '}')) {
i++;
continue; // 匹配成功,继续处理下一个字符
} else {
return false; // 括号不匹配,返回false
}
} else {
return false; // 栈空,右括号多余,返回false
}
}
i++;
}
return IsEmpty(S); // 最后判断栈是否为空,如果栈为空,则括号匹配成功,返回true;否则返回false
}
int main() {
Stack S;
InitStack(S);
char e;
int n;
cout << "请输入元素个数:" << endl;
cin >> n;
cout << "请依次输入栈的元素:" << endl;
for (int i = 0; i < n; i++) {
cin >> e;
Push(S, e);
}
cout << "栈中的元素为:";
while (!IsEmpty(S)) {
Pop(S, e);
cout << e << " ";
}
cout << endl;
char str[MAXSIZE];
cout << "请输入括号序列:" << endl;
cin >> str;
if (Match(str)) {
cout << "括号匹配成功!" << endl;
} else {
cout << "括号不匹配!" << endl;
}
return 0;
}
```
以上是实现顺序栈和括号匹配的代码,你可以通过输入元素和括号序列来进行测试。
阅读全文
相关推荐
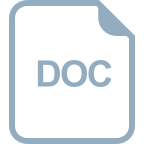
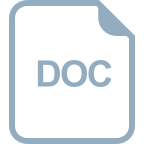
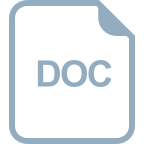

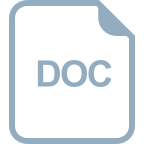
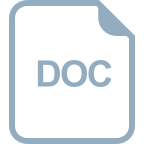
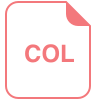
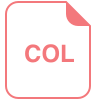
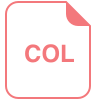
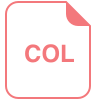
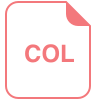
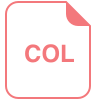
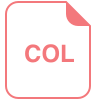
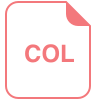
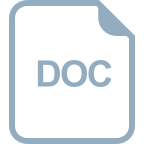
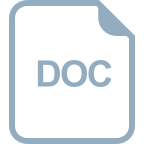
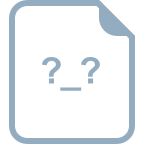
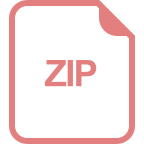