c语言程序 canopen pdo
时间: 2024-06-15 18:09:22 浏览: 93
CANopen是一种基于CAN总线的通信协议,用于在嵌入式系统中实现设备之间的通信。PDO(Process Data Object)是CANopen协议中的一种数据传输方式,用于实时传输设备之间的数据。
在C语言中,可以使用CANopen协议库来实现CANopen通信和PDO数据传输。以下是一个简单的C语言程序示例,用于发送和接收PDO数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <canopen.h> // CANopen协议库头文件
int main() {
// 初始化CANopen协议库
if (canopen_init() != 0) {
printf("CANopen协议库初始化失败\n");
return -1;
}
// 打开CAN总线设备
if (canopen_open("/dev/can0") != 0) {
printf("打开CAN总线设备失败\n");
return -1;
}
// 设置PDO通信参数
uint8_t node_id = 1; // 设备节点ID
uint16_t pdo_index = 0x2000; // PDO对象索引
uint8_t pdo_subindex = 1; // PDO对象子索引
// 发送PDO数据
uint8_t pdo_data[8] = {0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08}; // 要发送的PDO数据
if (canopen_send_pdo(node_id, pdo_index, pdo_subindex, pdo_data, 8) != 0) {
printf("发送PDO数据失败\n");
return -1;
}
// 接收PDO数据
uint8_t received_pdo_data[8];
if (canopen_receive_pdo(node_id, pdo_index, pdo_subindex, received_pdo_data, 8) != 0) {
printf("接收PDO数据失败\n");
return -1;
}
// 打印接收到的PDO数据
printf("接收到的PDO数据:");
for (int i = 0; i < 8; i++) {
printf("%02X ", received_pdo_data[i]);
}
printf("\n");
// 关闭CAN总线设备
canopen_close();
return 0;
}
```
这个程序示例演示了如何使用CANopen协议库在C语言中实现CANopen通信和PDO数据传输。你可以根据实际需求修改程序中的参数和数据。
相关推荐
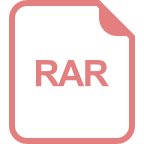














