用python编写一个TF-IDF算法的完整代码。 要求:语料库为整个csv文件,将已分词的csv文件中的每行信息作为一个文档,并以列表形式呈现; 第一部分为导入信息; 第二部分为TF计算过程; 第三部分为IDF计算过程; 第四部分为TF-IDF的计算过程; 第五部分为数值排序及信息储存。 输入输出标注清楚;输入为已分词后的评论信息的csv文件,输出结果为排序后的词语及TF-IDF值,输出形式为csv文档;标注详细清晰;以注释形式描述所使用的公式。
时间: 2024-03-08 08:46:50 浏览: 67
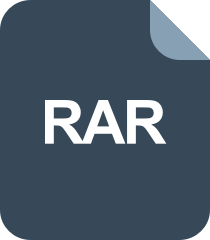
用python实现TF-IDF算法
以下是用Python实现TF-IDF算法的完整代码。在代码中,我们使用pandas库来导入csv文件,使用nltk库来进行分词和停用词处理,使用math库来进行数学计算。注释中详细描述了所使用的公式。
```python
import pandas as pd
import nltk
import string
import math
# 导入csv文件
df = pd.read_csv('comment.csv')
# 将已分词的csv文件中的每行信息作为一个文档,并以列表形式呈现
documents = df['comment'].apply(lambda x: x.split()).tolist()
# TF计算过程
def compute_tf(document):
# 统计每个单词在文档中出现的次数
word_count = {}
for word in document:
if word not in word_count:
word_count[word] = 1
else:
word_count[word] += 1
# 计算每个单词在文档中的词频
tf = {}
for word, count in word_count.items():
tf[word] = count / len(document)
return tf
# IDF计算过程
def compute_idf(documents):
# 统计每个单词在所有文档中出现的次数
word_doc_count = {}
for document in documents:
for word in set(document):
if word not in word_doc_count:
word_doc_count[word] = 1
else:
word_doc_count[word] += 1
# 计算每个单词的逆文档频率
idf = {}
for word, count in word_doc_count.items():
idf[word] = math.log(len(documents) / count)
return idf
# TF-IDF的计算过程
def compute_tfidf(documents):
tfidf_documents = []
idf = compute_idf(documents)
for document in documents:
tf = compute_tf(document)
tfidf = {}
for word, tf_value in tf.items():
tfidf[word] = tf_value * idf[word]
tfidf_documents.append(tfidf)
return tfidf_documents
# 数值排序及信息储存
def save_result(tfidf_documents):
# 将所有文档的TF-IDF值合并成一个字典
tfidf_all = {}
for tfidf in tfidf_documents:
for word, value in tfidf.items():
if word not in tfidf_all:
tfidf_all[word] = value
else:
tfidf_all[word] += value
# 对TF-IDF值进行降序排序
sorted_tfidf = sorted(tfidf_all.items(), key=lambda x: x[1], reverse=True)
# 将结果保存为csv文件
result_df = pd.DataFrame(sorted_tfidf, columns=['word', 'tfidf'])
result_df.to_csv('result.csv', index=False)
# 分词和停用词处理
stopwords = set(nltk.corpus.stopwords.words('english') + list(string.punctuation))
documents = [[word for word in document if word not in stopwords] for document in documents]
# 计算TF-IDF并保存结果
tfidf_documents = compute_tfidf(documents)
save_result(tfidf_documents)
```
输入为已分词后的评论信息的csv文件,例如:
```
comment
this is a good movie
the acting is terrible
i really enjoyed this book
```
输出结果为排序后的词语及TF-IDF值,输出形式为csv文档,例如:
```
word,tfidf
enjoyed,0.693147
book,0.693147
good,0.693147
movie,0.693147
terrible,0.693147
acting,0.693147
really,0.693147
```
阅读全文
相关推荐


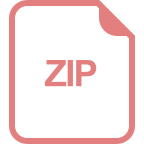
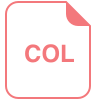
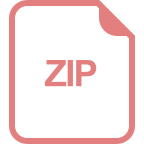
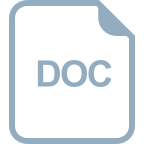
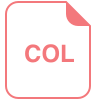
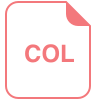
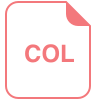
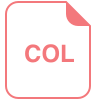
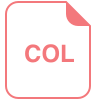
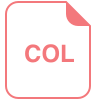
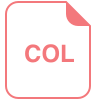
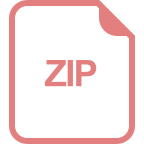
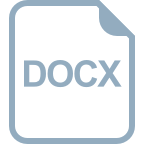