卷积神经网络实现手写数字图像识别 代码中需包括准确率图像
时间: 2023-10-11 12:13:07 浏览: 69
好的,关于您的问题,我可以简单的回答一下。手写数字图像识别是计算机视觉领域中的一个经典问题,其中卷积神经网络(Convolutional Neural Network,CNN)是实现这一任务的常用算法。以下是一个简单的CNN手写数字图像识别的代码示例,其中包括准确率图像的绘制。
```python
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
# 加载MNIST数据集
mnist = tf.keras.datasets.mnist
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
# 对图像进行预处理
train_images = train_images / 255.0
test_images = test_images / 255.0
# 构建CNN模型
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10)
])
# 编译模型
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# 训练模型
history = model.fit(train_images[..., np.newaxis], train_labels, epochs=10,
validation_data=(test_images[..., np.newaxis], test_labels))
# 绘制准确率图像
plt.plot(history.history['accuracy'], label='accuracy')
plt.plot(history.history['val_accuracy'], label = 'val_accuracy')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.ylim([0.5, 1])
plt.legend(loc='lower right')
plt.show()
```
在上述代码中,我们首先加载了MNIST数据集,并对图像进行了预处理。接着,我们构建了一个包含卷积层、池化层和全连接层的CNN模型,并编译该模型。随后,我们使用训练数据训练了该模型,并通过`history`变量获取了训练过程中的准确率数据。最后,我们使用Matplotlib库绘制了准确率图像,其中横轴为训练轮数,纵轴为准确率。
希望这个简单的代码示例可以回答您的问题。如果您有任何疑问,请随时向我提问。
相关推荐
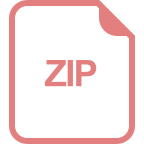
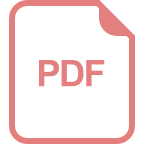
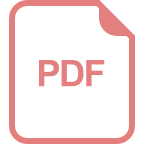














