Python俄罗斯方块
时间: 2023-11-29 13:46:20 浏览: 48
Python俄罗斯方块是一款基于Python语言开发的小游戏,玩家需要控制方块的移动和旋转,使其落到底部并填满一行或多行,以此获得分数。以下是Python俄罗斯方块的基本玩法和实现方式:
1.基本玩法:
- 游戏开始时,屏幕上会出现一个空白的游戏区域,玩家需要控制方块的移动和旋转,使其落到底部并填满一行或多行,以此获得分数。
- 方块的形状和颜色是随机生成的,玩家需要根据方块的形状和颜色来判断如何移动和旋转。
- 当方块落到底部时,如果一行或多行被填满,则该行将被消除,并获得相应的分数。
- 游戏结束的条件是游戏区域被填满,无法再放置新的方块。
2.实现方式:
- 可以使用Python的pygame库来实现游戏界面和交互逻辑。
- 游戏区域可以使用一个二维数组来表示,每个元素表示一个方块的状态(是否被占据、颜色等)。
- 方块的形状可以使用一个二维数组来表示,每个元素表示方块的一个小方块的状态(是否存在、颜色等)。
- 方块的移动和旋转可以通过修改方块的位置和形状来实现。
- 消除行可以通过遍历游戏区域,判断每一行是否被填满,然后将该行以上的所有行下移一行来实现。
- 分数可以通过消除行的数量来计算。
相关问题
python 俄罗斯方块
Python 俄罗斯方块可以使用 Pygame 模块来实现游戏的绘制和交互。具体实现步骤如下:
1. 导入 Pygame 模块
```python
import pygame
```
2. 初始化 Pygame
```python
pygame.init()
```
3. 设置游戏窗口大小和标题
```python
size = (800, 600)
screen = pygame.display.set_mode(size)
pygame.display.set_caption("俄罗斯方块")
```
4. 定义方块类和方块组类
```python
class Block(pygame.sprite.Sprite):
def __init__(self, color, width, height):
super().__init__()
self.image = pygame.Surface([width, height])
self.image.fill(color)
self.rect = self.image.get_rect()
class BlockGroup(pygame.sprite.Group):
def __init__(self):
super().__init__()
self.blocks = []
```
5. 定义游戏循环
```python
def game_loop():
block_group = BlockGroup()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
# 方块向左移动
elif event.key == pygame.K_RIGHT:
# 方块向右移动
elif event.key == pygame.K_DOWN:
# 方块加速下落
elif event.key == pygame.K_SPACE:
# 方块旋转
screen.fill((255, 255, 255))
block_group.draw(screen)
pygame.display.flip()
game_loop()
```
以上是 Python 俄罗斯方块的基本实现步骤,具体细节可以根据实际情况进行调整和完善。
python俄罗斯方块毕设
以下是关于Python俄罗斯方块毕设的介绍:
Python俄罗斯方块毕设是一种基于Python语言编写的俄罗斯方块游戏,可以作为毕业设计项目来完成。该项目需要使用Pygame模块,这是一系列专门为编写电子游戏而设计的Python模块,它在已经非常优秀的SDL库的基础上增加了许多功能,让你能够用Python语言编写出丰富多彩的游戏程序。
在实现Python俄罗斯方块毕设时,你需要掌握以下技能:
1. Pygame模块的使用,包括游戏窗口的创建、游戏场景的绘制、游戏音效的播放等。
2. 俄罗斯方块游戏的规则和玩法,包括方块的移动、旋转、消除等。
3. Python语言的基础知识,包括变量、函数、条件语句、循环语句等。
以下是一个简单的Python俄罗斯方块游戏的代码示例,供参考:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
WINDOW_SIZE = (WINDOW_WIDTH, WINDOW_HEIGHT)
# 设置游戏场景大小
SCENE_WIDTH = 300
SCENE_HEIGHT = 600
SCENE_SIZE = (SCENE_WIDTH, SCENE_HEIGHT)
# 设置游戏窗口标题
pygame.display.set_caption('Python俄罗斯方块')
# 创建游戏窗口
screen = pygame.display.set_mode(WINDOW_SIZE)
# 加载游戏音效
pygame.mixer.music.load('bgm.mp3')
pygame.mixer.music.play(-1)
# 定义方块的形状
shapes = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]],
[[7, 7, 7, 7]]
]
# 定义方块的颜色
colors = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(128, 128, 128)
]
# 定义方块的大小
BLOCK_SIZE = 30
# 定义游戏场景
scene = [[0] * (SCENE_WIDTH // BLOCK_SIZE) for _ in range(SCENE_HEIGHT // BLOCK_SIZE)]
# 定义当前方块
current_shape = random.choice(shapes)
current_color = random.randint(1, len(colors) - 1)
current_x = SCENE_WIDTH // BLOCK_SIZE // 2 - len(current_shape[0]) // 2
current_y = 0
# 定义游戏循环
clock = pygame.time.Clock()
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 绘制游戏场景
screen.fill((255, 255, 255))
for y, row in enumerate(scene):
for x, block in enumerate(row):
if block > 0:
pygame.draw.rect(screen, colors[block], (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制当前方块
for y, row in enumerate(current_shape):
for x, block in enumerate(row):
if block > 0:
pygame.draw.rect(screen, colors[current_color], ((current_x + x) * BLOCK_SIZE, (current_y + y) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 更新游戏场景
if pygame.time.get_ticks() % 500 == 0:
if current_y + len(current_shape) < len(scene) and not any([scene[current_y + y][current_x + x] > 0 for y, row in enumerate(current_shape) for x, block in enumerate(row) if block > 0]):
current_y += 1
else:
for y, row in enumerate(current_shape):
for x, block in enumerate(row):
if block > 0:
scene[current_y + y][current_x + x] = current_color
current_shape = random.choice(shapes)
current_color = random.randint(1, len(colors) - 1)
current_x = SCENE_WIDTH // BLOCK_SIZE // 2 - len(current_shape[0]) // 2
current_y = 0
# 更新游戏窗口
pygame.display.update()
# 控制游戏帧率
clock.tick(60)
```
相关推荐
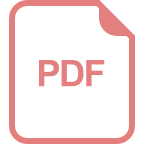






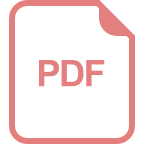
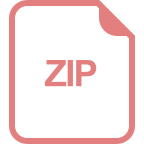