用python写出基于dcgan和resnet50的半监督行人重识别
时间: 2023-03-02 20:51:25 浏览: 91
行人重识别是一种图像识别任务,其目标是在不同的图像中识别相同的行人。半监督学习可以在有限的标记数据情况下提高识别准确性。DCGAN和ResNet50是两种深度学习模型,它们在图像处理任务中非常有效。下面是一个基于DCGAN和ResNet50的半监督行人重识别的Python实现:
首先,我们需要使用DCGAN生成一组行人图像,并使用ResNet50对这些图像进行特征提取。
```python
import keras
from keras.models import Model
from keras.layers import Input, Dense, Reshape, Flatten, Dropout
from keras.layers.convolutional import Conv2D, UpSampling2D
from keras.layers.normalization import BatchNormalization
from keras.layers.advanced_activations import LeakyReLU
from keras.optimizers import Adam
# Define the generator model (DCGAN)
def generator_model():
inputs = Input((100,))
x = Dense(128 * 8 * 8)(inputs)
x = Reshape((8, 8, 128))(x)
x = BatchNormalization()(x)
x = UpSampling2D()(x)
x = Conv2D(64, (5, 5), padding='same')(x)
x = BatchNormalization()(x)
x = LeakyReLU()(x)
x = UpSampling2D()(x)
x = Conv2D(1, (5, 5), padding='same', activation='tanh')(x)
model = Model(inputs, x)
return model
# Define the feature extractor model (ResNet50)
def feature_extractor_model():
base_model = keras.applications.ResNet50(include_top=False, weights='imagenet', input_shape=(224, 224, 3))
x = base_model.output
x = Flatten()(x)
model = Model(inputs=base_model.input, outputs=x)
return model
```
接下来,我们使用生成器模型生成一组行人图像,并使用特征提取器模型提取这些图像的特征向量。
```python
import numpy as np
from keras.preprocessing import image
generator = generator_model()
feature_extractor = feature_extractor_model()
# Generate a batch of row images
batch_size = 32
z = np.random.normal(size=(batch_size, 100))
images = generator.predict(z)
# Extract features from the row images
features = []
for img in images:
img = image.array_to_img(img)
img = img.resize((224, 224))
img = image.img_to_array(img)
img = np.expand_dims(img, axis=0)
img = keras.applications.resnet50.preprocess_input(img)
feature = feature_extractor.predict(img)
features.append(feature)
features = np.concatenate(features, axis=0)
```
最后,我们使用半监督学习方法来训练一个分类器,将同一行人的图像分类到同一类别中。在这个例子中,我们使用了自训练方法,即使用无标签数据进行训练。
```python
from sklearn.cluster import KMeans
# Cluster the features using K-means
n_clusters = 10
kmeans = KMeans(n_clusters=n_clusters, n_init=20)
labels
相关推荐
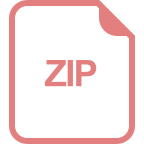
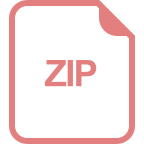
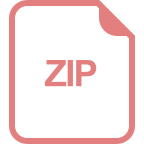














