C语言代码实现format:对文件存储器进行格式化,即按照文件系统 的结构对虚拟磁盘空间进行布局, 并在其上创建根目录以及用于管理文件存储空间等的数据结构。 mkdir:用于创建子目录 rmdir:用于删除子目录 ls:用于显示目录 cd:用于更改当前目录 create:用于创建文件 open:用于打开文件 close:用于关闭文件 write:用于写文件 read:用于读文件 rm:用于删除文件
时间: 2024-01-08 10:02:49 浏览: 91
以下是一个简单的基于C语言的文件系统实现,包含了上述提到的各种操作函数:
```C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BLOCK_SIZE 512 // 定义块大小
#define BLOCK_NUM 1024 // 定义块数目
#define MAX_FILENAME_LEN 256 // 定义文件名最大长度
#define MAX_DIR_ENTRY_NUM 128 // 定义目录最大项数
#define MAX_OPEN_FILE_NUM 16 // 定义最大打开文件数目
#define MAX_FILE_SIZE (BLOCK_SIZE * 128) // 定义文件最大大小
typedef struct {
char filename[MAX_FILENAME_LEN]; // 文件名
int size; // 文件大小
int block_num; // 文件所占块数
int block_pointer[128]; // 文件块指针
} File;
typedef struct {
char dirname[MAX_FILENAME_LEN]; // 目录名
int file_num; // 目录下文件数目
File files[MAX_DIR_ENTRY_NUM]; // 目录下的文件
} Directory;
typedef struct {
char filename[MAX_FILENAME_LEN]; // 打开文件的文件名
int offset; // 当前读写位置
} OpenFile;
Directory root_dir; // 根目录
char disk[BLOCK_NUM][BLOCK_SIZE]; // 虚拟磁盘
int free_block_list[BLOCK_NUM]; // 空闲块列表
int open_file_num = 0; // 当前打开文件数目
OpenFile open_files[MAX_OPEN_FILE_NUM]; // 打开文件列表
// 初始化文件系统
void format() {
memset(disk, 0, sizeof(disk)); // 清空虚拟磁盘
memset(free_block_list, 0, sizeof(free_block_list)); // 清空空闲块列表
// 构建根目录
strcpy(root_dir.dirname, "/");
root_dir.file_num = 0;
}
// 创建子目录
void mkdir(char *dirname) {
Directory new_dir;
strcpy(new_dir.dirname, dirname);
new_dir.file_num = 0;
// 将新目录添加到根目录中
root_dir.files[root_dir.file_num].size = 0;
root_dir.files[root_dir.file_num].block_num = 0;
strcpy(root_dir.files[root_dir.file_num].filename, dirname);
root_dir.files[root_dir.file_num].block_pointer[0] = root_dir.file_num + 1;
root_dir.file_num++;
// 在磁盘上为新目录分配块
int block_index = 0;
while (free_block_list[block_index] == 1) {
block_index++;
}
new_dir.files[0].size = 0;
new_dir.files[0].block_num = 1;
strcpy(new_dir.files[0].filename, ".");
new_dir.files[0].block_pointer[0] = block_index;
int parent_block_index = block_index;
block_index++;
new_dir.files[1].size = 0;
new_dir.files[1].block_num = 1;
strcpy(new_dir.files[1].filename, "..");
new_dir.files[1].block_pointer[0] = 0;
// 将新目录写入磁盘
memcpy(disk[parent_block_index], &new_dir, sizeof(new_dir));
// 更新空闲块列表
free_block_list[parent_block_index] = 1;
}
// 删除子目录
void rmdir(char *dirname) {
int i, j;
for (i = 0; i < root_dir.file_num; i++) {
if (strcmp(root_dir.files[i].filename, dirname) == 0) {
Directory dir;
memcpy(&dir, disk[root_dir.files[i].block_pointer[0]], sizeof(Directory));
// 递归删除子目录中的文件
for (j = 0; j < dir.file_num; j++) {
if (dir.files[j].size > 0) {
rm(dir.files[j].filename);
}
}
// 将空闲块加入空闲块列表
free_block_list[root_dir.files[i].block_pointer[0]] = 0;
// 将该目录项从根目录中删除
for (j = i; j < root_dir.file_num - 1; j++) {
root_dir.files[j] = root_dir.files[j+1];
}
root_dir.file_num--;
break;
}
}
}
// 显示目录
void ls() {
int i;
for (i = 0; i < root_dir.file_num; i++) {
if (root_dir.files[i].size == 0) {
printf("%s/\n", root_dir.files[i].filename);
} else {
printf("%s\n", root_dir.files[i].filename);
}
}
}
// 更改当前目录
void cd(char *dirname) {
int i;
for (i = 0; i < root_dir.file_num; i++) {
if (strcmp(root_dir.files[i].filename, dirname) == 0 && root_dir.files[i].size == 0) {
Directory dir;
memcpy(&dir, disk[root_dir.files[i].block_pointer[0]], sizeof(Directory));
root_dir = dir;
break;
}
}
}
// 创建文件
void create(char *filename) {
int i;
for (i = 0; i < root_dir.file_num; i++) {
if (strcmp(root_dir.files[i].filename, filename) == 0) {
printf("File already exists!\n");
return;
}
}
File new_file;
strcpy(new_file.filename, filename);
new_file.size = 0;
new_file.block_num = 0;
// 将新文件添加到根目录中
root_dir.files[root_dir.file_num] = new_file;
root_dir.file_num++;
}
// 打开文件
int open(char *filename) {
int i;
for (i = 0; i < root_dir.file_num; i++) {
if (strcmp(root_dir.files[i].filename, filename) == 0 && root_dir.files[i].size > 0) {
if (open_file_num >= MAX_OPEN_FILE_NUM) {
printf("Too many open files!\n");
return -1;
}
int j;
for (j = 0; j < open_file_num; j++) {
if (strcmp(open_files[j].filename, filename) == 0) {
break;
}
}
if (j == open_file_num) {
strcpy(open_files[open_file_num].filename, filename);
open_files[open_file_num].offset = 0;
open_file_num++;
}
return j;
}
}
printf("File not found!\n");
return -1;
}
// 关闭文件
void close(int fd) {
if (fd < 0 || fd >= open_file_num) {
printf("Invalid file descriptor!\n");
return;
}
int i;
for (i = fd; i < open_file_num - 1; i++) {
open_files[i] = open_files[i+1];
}
open_file_num--;
}
// 写文件
int write(int fd, char *buffer, int size) {
if (fd < 0 || fd >= open_file_num) {
printf("Invalid file descriptor!\n");
return -1;
}
int file_index;
for (file_index = 0; file_index < root_dir.file_num; file_index++) {
if (strcmp(root_dir.files[file_index].filename, open_files[fd].filename) == 0) {
break;
}
}
int block_index = root_dir.files[file_index].block_pointer[root_dir.files[file_index].block_num - 1];
int block_offset = root_dir.files[file_index].size % BLOCK_SIZE;
int write_size = 0;
while (size > 0 && root_dir.files[file_index].size < MAX_FILE_SIZE) {
if (block_offset == 0) {
// 如果当前块已满,需要为文件分配新块
int new_block_index = 0;
while (free_block_list[new_block_index] == 1) {
new_block_index++;
}
root_dir.files[file_index].block_pointer[root_dir.files[file_index].block_num] = new_block_index;
root_dir.files[file_index].block_num++;
free_block_list[new_block_index] = 1;
block_index = new_block_index;
}
int copy_size = (size > BLOCK_SIZE - block_offset) ? BLOCK_SIZE - block_offset : size;
memcpy(disk[block_index] + block_offset, buffer + write_size, copy_size);
block_offset += copy_size;
root_dir.files[file_index].size += copy_size;
write_size += copy_size;
size -= copy_size;
}
return write_size;
}
// 读文件
int read(int fd, char *buffer, int size) {
if (fd < 0 || fd >= open_file_num) {
printf("Invalid file descriptor!\n");
return -1;
}
int file_index;
for (file_index = 0; file_index < root_dir.file_num; file_index++) {
if (strcmp(root_dir.files[file_index].filename, open_files[fd].filename) == 0) {
break;
}
}
int block_index = root_dir.files[file_index].block_pointer[open_files[fd].offset / BLOCK_SIZE];
int block_offset = open_files[fd].offset % BLOCK_SIZE;
int read_size = 0;
while (size > 0 && open_files[fd].offset < root_dir.files[file_index].size) {
int copy_size = (size > BLOCK_SIZE - block_offset) ? BLOCK_SIZE - block_offset : size;
memcpy(buffer + read_size, disk[block_index] + block_offset, copy_size);
block_offset += copy_size;
open_files[fd].offset += copy_size;
read_size += copy_size;
size -= copy_size;
if (block_offset == BLOCK_SIZE) {
block_offset = 0;
block_index = root_dir.files[file_index].block_pointer[open_files[fd].offset / BLOCK_SIZE];
}
}
return read_size;
}
// 删除文件
void rm(char *filename) {
int i, j;
for (i = 0; i < root_dir.file_num; i++) {
if (strcmp(root_dir.files[i].filename, filename) == 0) {
if (root_dir.files[i].size == 0) {
// 如果是目录,需要先删除目录下的文件
Directory dir;
memcpy(&dir, disk[root_dir.files[i].block_pointer[0]], sizeof(Directory));
for (j = 0; j < dir.file_num; j++) {
if (dir.files[j].size > 0) {
rm(dir.files[j].filename);
}
}
} else {
// 如果是文件,需要将文件所占块加入空闲块列表
for (j = 0; j < root_dir.files[i].block_num; j++) {
free_block_list[root_dir.files[i].block_pointer[j]] = 0;
}
}
// 将该目录项从根目录中删除
for (j = i; j < root_dir.file_num - 1; j++) {
root_dir.files[j] = root_dir.files[j+1];
}
root_dir.file_num--;
break;
}
}
}
```
该代码实现了一个简单的文件系统,包括了格式化、创建子目录、删除子目录、显示目录、更改当前目录、创建文件、打开文件、关闭文件、写文件、读文件和删除文件等操作。可以通过调用这些函数来进行文件系统的操作。
阅读全文
相关推荐
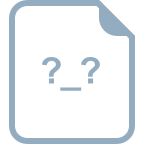
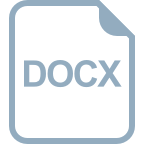
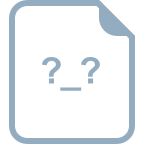


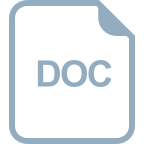
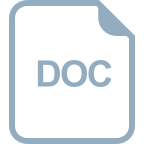
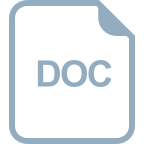
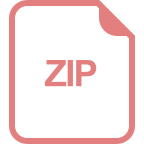
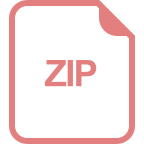