python实现计算文件中的英语单词的熵
时间: 2024-05-10 14:21:17 浏览: 11
熵是一个概率分布的不确定性度量,可以通过以下步骤计算一个文件中英语单词的熵:
1. 读取文件内容并转换为小写字母,去除标点符号和数字,只保留单词。
2. 统计每个单词的出现次数,并计算每个单词的概率。
3. 计算每个单词的信息量,即-log2(p),其中p为单词出现的概率。
4. 将每个单词的信息量乘以其概率,得到每个单词对熵的贡献。
5. 将所有单词对熵的贡献相加,得到文件中英语单词的熵。
下面是一个Python实现的示例代码:
```python
import re
import math
def calculate_entropy(file_path):
# 读取文件内容并转换为小写字母
with open(file_path, 'r') as f:
content = f.read().lower()
# 去除标点符号和数字,只保留单词
words = re.findall(r'\b\w+\b', content)
# 统计每个单词的出现次数
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 计算每个单词的概率
total_words = len(words)
word_prob = {}
for word, count in word_count.items():
word_prob[word] = count / total_words
# 计算每个单词的信息量,并乘以其概率
word_entropy = {}
for word, prob in word_prob.items():
word_entropy[word] = -math.log2(prob) * prob
# 将所有单词对熵的贡献相加,得到文件中英语单词的熵
entropy = sum(word_entropy.values())
return entropy
```
使用示例:
```python
file_path = 'example.txt'
entropy = calculate_entropy(file_path)
print('Entropy:', entropy)
```
其中example.txt为要计算熵的文件路径。
相关推荐
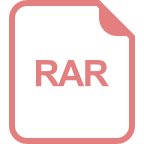
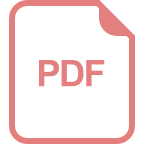
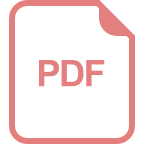
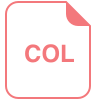
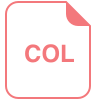
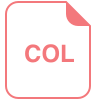
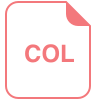
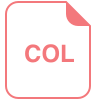









