public interface IState { void Enter(); void Update(); void Exit(); } public class IdleState : IState { public void Enter() { // Do idle behavior } public void Update() { // Do idle behavior } public void Exit() { // Do idle behavior } } public class WalkingState : IState { public void Enter() { // Do walking behavior } public void Update() { // Do walking behavior } public void Exit() { // Do walking behavior } } public class RunningState : IState { public void Enter() { // Do running behavior } public void Update() { // Do running behavior } public void Exit() { // Do running behavior } } public class StateMachine<T> where T : IState { private T currentState; public void SetState(T newState) { if (currentState != null) { currentState.Exit(); } currentState = newState; currentState.Enter(); } public void Update() { if (currentState != null) { currentState.Update(); } } }
时间: 2023-04-05 13:03:39 浏览: 97
这是一个关于状态机的代码实现,其中定义了一个接口 IState,以及三个实现了该接口的类 IdleState、WalkingState 和 RunningState。另外还有一个泛型类 StateMachine,用于管理状态的转换和更新。在该类中,通过 SetState 方法可以切换当前状态,而 Update 方法则用于更新当前状态的行为。
相关问题
public interface IState { void Enter(); void Update(); void Exit(); } public class State<T> where T : IState { public delegate void StateAction(T state); public StateAction OnEnter; public StateAction OnUpdate; public StateAction OnExit; public void Enter(T state) { if (OnEnter != null) { OnEnter(state); } } public void Update(T state) { if (OnUpdate != null) { OnUpdate(state); } } public void Exit(T state) { if (OnExit != null) { OnExit(state); } } } public class IdleState : IState { public void Enter() { // Do idle behavior } public void Update() { // Do idle behavior } public void Exit() { // Do idle behavior } } public class WalkingState : IState { public void Enter() { // Do walking behavior } public void Update() { // Do walking behavior } public void Exit() { // Do walking behavior } } public class RunningState : IState { public void Enter() { // Do running behavior } public void Update() { // Do running behavior } public void Exit() { // Do running behavior } } public class StateMachine<T> where T : IState { private Stack<State<T>> stateStack = new Stack<State<T>>(); private T currentState; public void PushState(State<T> newState) { if (currentState != null) { currentState.Exit(); } stateStack.Push(newState); currentState = newState; currentState.Enter(currentState); } public void PopState() { if (stateStack.Count > 0) { currentState.Exit(); stateStack.Pop(); currentState = stateStack.Count > 0 ? stateStack.Peek() : null; if (currentState != null) { currentState.Enter(currentState); } } } public void Update() { if (currentState != null) { currentState.Update(currentState); } } }如何使用,请给出示例代码
这是一个状态机的实现,可以用于游戏开发等领域。使用方法如下:
1.定义状态类,实现IState接口,重写Enter、Update、Exit方法,分别表示进入状态、更新状态、退出状态时的行为。
2.创建StateMachine对象,指定泛型参数为状态类。
3.通过PushState方法将初始状态压入状态栈。
4.在游戏循环中,调用Update方法更新当前状态。
5.根据游戏逻辑,通过PushState和PopState方法切换状态。
示例代码如下:
IdleState idleState = new IdleState();
WalkingState walkingState = new WalkingState();
RunningState runningState = new RunningState();
StateMachine<IState> stateMachine = new StateMachine<IState>();
stateMachine.PushState(idleState);
while (true) {
stateMachine.Update();
// 根据游戏逻辑切换状态
if (playerInput == "walk") {
stateMachine.PushState(walkingState);
} else if (playerInput == "run") {
stateMachine.PushState(runningState);
} else if (playerInput == "stop") {
stateMachine.PopState();
}
}
public interface IState { string Name { get; } Action EnterAction { get; } Action UpdateAction { get; } Action ExitAction { get; } } public class StateMachine<T> where T : IState { private T currentState; private Dictionary<string, IState> stateDict = new Dictionary<string, IState>(); private float updateInterval = 0.1f; private float updateTimer = 0f; public void AddState(T state) { stateDict[state.Name] = state; } public void SetState(string stateName) { if (currentState != null) { currentState.ExitAction(); } currentState = (T)stateDict[stateName]; currentState.EnterAction(); } public void Update() { updateTimer += Time.deltaTime; if (updateTimer >= updateInterval) { if (currentState != null) { currentState.UpdateAction(); } updateTimer = 0f; } } } public class IdleState : IState { public string Name { get { return "Idle"; } } public Action EnterAction { get { return () => { /* Do idle behavior */ }; } } public Action UpdateAction { get { return () => { /* Do idle behavior */ }; } } public Action ExitAction { get { return () => { /* Do idle behavior */ }; } } } public class WalkingState : IState { public string Name { get { return "Walking"; } } public Action EnterAction { get { return () => { /* Do walking behavior */ }; } } public Action UpdateAction { get { return () => { /* Do walking behavior */ }; } } public Action ExitAction { get { return () => { /* Do walking behavior */ }; } } } public class RunningState : IState { public string Name { get { return "Running"; } } public Action EnterAction { get { return () => { /* Do running behavior */ }; } } public Action UpdateAction { get { return () => { /* Do running behavior */ }; } } public Action ExitAction { get { return () => { /* Do running behavior */ }; } } }如何使用
这是一个状态机的示例代码,可以用于控制游戏角色的行为。首先需要定义不同的状态,例如IdleState、WalkingState和RunningState,每个状态都实现了IState接口,包括Name、EnterAction、UpdateAction和ExitAction四个属性或方法。然后可以创建一个StateMachine<T>对象,其中T是状态的类型,通过AddState方法添加不同的状态,通过SetState方法设置当前状态,通过Update方法更新状态。在每个状态的EnterAction、UpdateAction和ExitAction方法中,可以编写具体的行为逻辑。
阅读全文
相关推荐
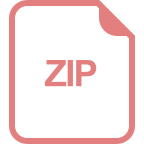
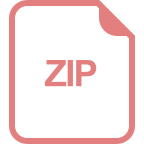
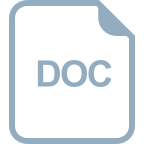
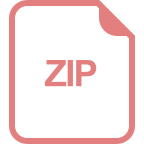
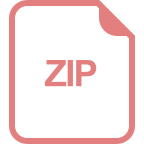
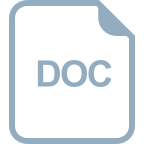
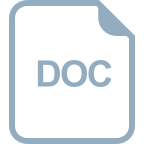
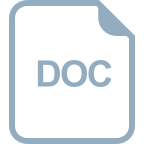





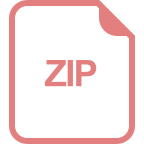
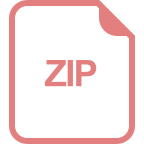