用c调用lzma压缩文件
时间: 2024-01-26 15:46:51 浏览: 173

lzma压缩算法源 c 代码

要在C语言中调用 LZMA 压缩文件,需要使用 LZMA SDK 提供的函数和结构体。以下是一个基本的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <lzma.h>
#define BUF_SIZE 1024
int main(int argc, char *argv[])
{
if (argc != 3) {
fprintf(stderr, "Usage: %s <source_file> <dest_file>\n", argv[0]);
exit(EXIT_FAILURE);
}
FILE *src_file = fopen(argv[1], "rb");
if (!src_file) {
fprintf(stderr, "Failed to open source file: %s\n", strerror(errno));
exit(EXIT_FAILURE);
}
FILE *dst_file = fopen(argv[2], "wb");
if (!dst_file) {
fprintf(stderr, "Failed to open destination file: %s\n", strerror(errno));
fclose(src_file);
exit(EXIT_FAILURE);
}
lzma_stream strm = LZMA_STREAM_INIT;
lzma_ret ret = lzma_easy_encoder(&strm, 6, LZMA_CHECK_CRC64);
if (ret != LZMA_OK) {
fprintf(stderr, "Failed to initialize LZMA encoder: %s\n", lzma_strerror(ret));
fclose(dst_file);
fclose(src_file);
exit(EXIT_FAILURE);
}
uint8_t in_buf[BUF_SIZE];
uint8_t out_buf[BUF_SIZE];
strm.next_out = out_buf;
strm.avail_out = sizeof(out_buf);
do {
strm.next_in = in_buf;
strm.avail_in = fread(in_buf, 1, sizeof(in_buf), src_file);
if (ferror(src_file)) {
fprintf(stderr, "Failed to read source file: %s\n", strerror(errno));
lzma_end(&strm);
fclose(dst_file);
fclose(src_file);
exit(EXIT_FAILURE);
}
ret = lzma_code(&strm, LZMA_RUN);
if (ret != LZMA_OK && ret != LZMA_STREAM_END) {
fprintf(stderr, "Failed to compress data: %s\n", lzma_strerror(ret));
lzma_end(&strm);
fclose(dst_file);
fclose(src_file);
exit(EXIT_FAILURE);
}
size_t out_size = sizeof(out_buf) - strm.avail_out;
if (fwrite(out_buf, 1, out_size, dst_file) != out_size) {
fprintf(stderr, "Failed to write compressed data: %s\n", strerror(errno));
lzma_end(&strm);
fclose(dst_file);
fclose(src_file);
exit(EXIT_FAILURE);
}
strm.next_out = out_buf;
strm.avail_out = sizeof(out_buf);
} while (strm.avail_in > 0);
ret = lzma_code(&strm, LZMA_FINISH);
if (ret != LZMA_OK && ret != LZMA_STREAM_END) {
fprintf(stderr, "Failed to finish compression: %s\n", lzma_strerror(ret));
lzma_end(&strm);
fclose(dst_file);
fclose(src_file);
exit(EXIT_FAILURE);
}
size_t out_size = sizeof(out_buf) - strm.avail_out;
if (fwrite(out_buf, 1, out_size, dst_file) != out_size) {
fprintf(stderr, "Failed to write compressed data: %s\n", strerror(errno));
lzma_end(&strm);
fclose(dst_file);
fclose(src_file);
exit(EXIT_FAILURE);
}
lzma_end(&strm);
fclose(dst_file);
fclose(src_file);
return 0;
}
```
这个程序会将源文件压缩为 LZMA 格式,并保存到目标文件中。在程序中,使用 `lzma_easy_encoder` 函数初始化 LZMA 编码器,使用 `lzma_code` 函数进行压缩,最后使用 `lzma_end` 函数关闭编码器。需要注意的是,LZMA SDK 提供了多种压缩选项,例如压缩级别和校验方式,在这个示例中使用的是较为常见的选项。
阅读全文
相关推荐
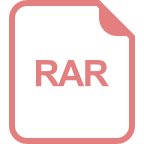
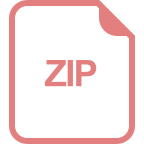


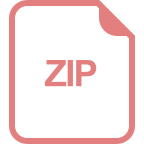
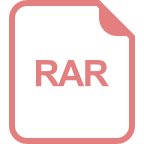
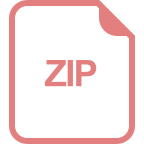

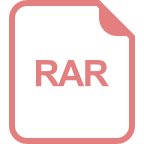
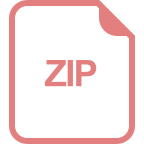
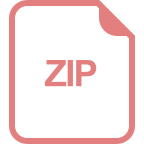
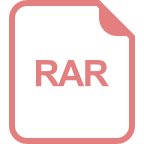
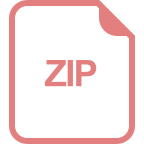
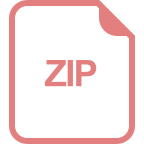
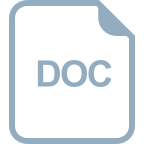
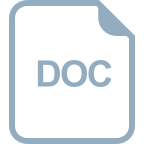
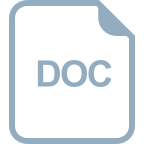
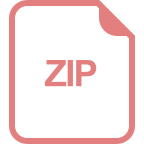