基于gym实现有风的格子世界
时间: 2023-12-11 14:01:54 浏览: 40
好的,这里是一个基于 `gym` 实现的有风格子世界的示例代码:
```python
import gym
from gym import spaces
import numpy as np
class WindyGridWorld(gym.Env):
def __init__(self):
# 定义动作空间和状态空间
self.action_space = spaces.Discrete(4) # 上下左右四个方向
self.observation_space = spaces.Box(low=0, high=1, shape=(7, 10), dtype=np.float32)
# 初始化状态和风力值
self.current_pos = (3, 0)
self.wind = [0, 0, 0, 1, 1, 1, 2, 2, 1, 0]
# 定义目标状态和奖励
self.goal_pos = (3, 7)
self.reward_goal = 0
self.reward_move = -1
self.reward_obstacle = -100
def reset(self):
# 初始化状态和风力值
self.current_pos = (3, 0)
self.wind = [0, 0, 0, 1, 1, 1, 2, 2, 1, 0]
return self._get_obs()
def step(self, action):
x, y = self.current_pos
wind = self.wind[y]
# 根据动作更新位置
if action == 0: # 上
x = max(0, x - 1 - wind)
elif action == 1: # 右
y = min(9, y + 1)
x = max(0, x - wind)
elif action == 2: # 下
x = min(6, x + 1 - wind)
elif action == 3: # 左
y = max(0, y - 1)
x = max(0, x - wind)
# 判断是否到达目标位置并给予相应奖励
if (x, y) == self.goal_pos:
reward = self.reward_goal
done = True
else:
reward = self.reward_move
done = False
# 判断是否撞到障碍物并给予相应奖励
if x == 3 and (1 <= y <= 8):
reward = self.reward_obstacle
# 更新状态
self.current_pos = (x, y)
return self._get_obs(), reward, done, {}
def _get_obs(self):
# 构造状态矩阵
obs = np.zeros((7, 10), dtype=np.float32)
obs[self.current_pos] = 1
return obs
```
在这个示例代码中,我们创建了一个名为 `WindyGridWorld` 的环境类,它继承了 `gym.Env` 类。在 `__init__` 方法中,我们定义了动作空间和状态空间。动作空间是一个离散的空间,包含四个动作:上下左右。状态空间是一个 $7 \times 10$ 的矩阵,表示格子世界的状态。
在 `reset` 方法中,我们初始化了初始状态和风力值,并返回初始状态。在 `step` 方法中,我们根据输入的动作更新位置,判断是否到达目标位置或撞到障碍物,并给予相应的奖励。最后更新状态并返回新的状态、奖励、是否完成和一些额外信息。在 `_get_obs` 方法中,我们构造了状态矩阵,表示当前位置。
相关推荐
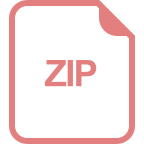














