用C++实现敏感词检测的后端
时间: 2023-06-17 10:03:39 浏览: 197
敏感词检测是一种文本过滤技术,可以通过对文本进行过滤来保护用户免受有害信息和不良言论的影响。下面是一个用C++实现敏感词检测的后端的示例代码:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <fstream>
using namespace std;
class TrieNode {
public:
TrieNode() {
for (int i = 0; i < 26; i++) {
next[i] = nullptr;
}
is_end = false;
}
TrieNode* next[26];
bool is_end;
};
class TrieTree {
public:
TrieTree() {
root = new TrieNode();
}
void insert(string word) {
TrieNode* p = root;
for (char c : word) {
if (p->next[c - 'a'] == nullptr) {
p->next[c - 'a'] = new TrieNode();
}
p = p->next[c - 'a'];
}
p->is_end = true;
}
bool search(string word) {
TrieNode* p = root;
for (char c : word) {
if (p->next[c - 'a'] == nullptr) {
return false;
}
p = p->next[c - 'a'];
}
return p->is_end;
}
bool startsWith(string prefix) {
TrieNode* p = root;
for (char c : prefix) {
if (p->next[c - 'a'] == nullptr) {
return false;
}
p = p->next[c - 'a'];
}
return true;
}
private:
TrieNode* root;
};
int main() {
TrieTree tree;
ifstream fin("sensitive_words.txt");
if (fin) {
string line;
while (getline(fin, line)) {
tree.insert(line);
}
fin.close();
}
string text;
cout << "请输入文本:" << endl;
getline(cin, text);
vector<string> sensitive_words;
for (int i = 0; i < text.length(); i++) {
for (int j = 1; j <= 10 && i + j <= text.length(); j++) {
string word = text.substr(i, j);
if (tree.search(word)) {
sensitive_words.push_back(word);
}
}
}
if (sensitive_words.empty()) {
cout << "文本中没有敏感词。" << endl;
} else {
cout << "文本中的敏感词有:" << endl;
for (string word : sensitive_words) {
cout << word << endl;
}
}
return 0;
}
```
上述代码主要使用了Trie树,通过将敏感词构建成Trie树,可以快速地进行检索。具体来说,首先从文件中读取敏感词并插入到Trie树中,然后对输入的文本进行遍历,每次取出一个长度为1到10之间的子串,在Trie树中查找是否存在该子串。如果存在,则将其添加到敏感词列表中。最后,输出敏感词列表即可。
阅读全文
相关推荐
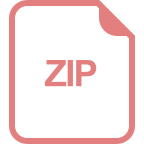
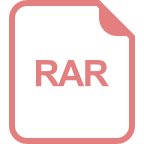
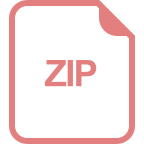















