android bottomsheet
时间: 2023-04-23 21:02:30 浏览: 246
Android BottomSheet是一种UI组件,通常用于显示应用程序中的菜单、操作或其他内容。它可以从屏幕底部滑出,并覆盖部分或全部屏幕。BottomSheet可以分为两种类型:模态和非模态。模态BottomSheet会阻止用户与应用程序的其他部分进行交互,而非模态BottomSheet则允许用户在打开的同时与应用程序的其他部分进行交互。BottomSheet通常与RecyclerView或ListView等列表视图一起使用,以便在底部显示滚动内容。
相关问题
BottomSheet 使用示例
下面是一个简单的BottomSheet使用示例:
1. 在XML布局文件中定义BottomSheet:
```xml
<LinearLayout
android:id="@+id/bottom_sheet"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:background="@android:color/white"
app:behavior_hideable="true"
app:behavior_peekHeight="50dp"
app:layout_behavior="com.google.android.material.bottomsheet.BottomSheetBehavior">
<!-- 在这里添加BottomSheet中的控件 -->
</LinearLayout>
```
2. 在代码中获取BottomSheetBehavior:
```java
LinearLayout bottomSheet = findViewById(R.id.bottom_sheet);
BottomSheetBehavior bottomSheetBehavior = BottomSheetBehavior.from(bottomSheet);
```
3. 设置BottomSheet的状态:
```java
// 显示BottomSheet
bottomSheetBehavior.setState(BottomSheetBehavior.STATE_EXPANDED);
// 隐藏BottomSheet
bottomSheetBehavior.setState(BottomSheetBehavior.STATE_HIDDEN);
```
4. 监听BottomSheet状态的变化:
```java
bottomSheetBehavior.setBottomSheetCallback(new BottomSheetBehavior.BottomSheetCallback() {
@Override
public void onStateChanged(@NonNull View bottomSheet, int newState) {
// 处理BottomSheet状态变化的逻辑
}
@Override
public void onSlide(@NonNull View bottomSheet, float slideOffset) {
// 处理BottomSheet滑动的逻辑
}
});
```
这就是一个简单的BottomSheet使用示例。你可以在BottomSheet中添加各种控件和信息,以满足你的应用程序的需求。
react native Bottom Sheet 使用
React Native 的 BottomSheet 是一个非常有用的组件,它允许你创建从屏幕底部弹出的可滑动视图,常用于展示附加信息、选项面板或模态对话框。以下是使用 React Native 底部抽屉(Bottom Sheet)的基本步骤:
1. 安装依赖:首先需要安装 `react-native-reanimated` 和 `react-native-gesture-handler` 来支持动画和手势处理。可以使用 npm 或 yarn 进行安装:
```bash
npm install react-native-reanimated react-native-gesture-handler@latest
```
然后,在项目配置文件 (如 AndroidManifest.xml) 中添加权限:
```xml
<uses-permission android:name="android.permission.SYSTEM_ALERT_WINDOW" />
```
2. 导入库和组件:在你的组件中导入必要的库和组件:
```javascript
import { View, StyleSheet, TouchableOpacity } from 'react-native';
import { PanGestureHandler, BottomSheet } from 'react-native-gesture-handler';
import Animated from 'react-native-reanimated';
```
3. 创建 BottomSheet 组件:
```javascript
const styles = StyleSheet.create({
bottomSheet: {
backgroundColor: 'white',
height: 0,
padding: 24,
borderRadius: 8,
},
});
function MyBottomSheet() {
const [sheetHeight, setSheetHeight] = useState(Animated.Value(0));
const onPanGestureEnd = () => {
if (sheetHeight.value > 50) {
setSheetHeight(new Animated.Value(-200));
} else {
setSheetHeight(new Animated.Value(0));
}
};
return (
<View style={styles.container}>
{/* 主体内容 */}
<TouchableOpacity
onPress={() => setSheetHeight(sheetHeight.value - 200)}
>
{/* 拖动开启 BottomSheet */}
</TouchableOpacity>
<PanGestureHandler onHandlerStateChange={(event) => {
if (event.state === 'active') {
sheetHeight.setValue(0);
}
}}>
<BottomSheet
ref={ref => (bottomSheetRef = ref)}
animatedValue={sheetHeight}
panGestureEnabled={true}
onGestureEnd={onPanGestureEnd}
sheetStyle={{
height: sheetHeight.interpolate({
inputRange: [0, -200],
outputRange: [0, 200], // 自定义最大高度
}),
}}
/>
</PanGestureHandler>
</View>
);
}
```
4. 使用 `<MyBottomSheet />` 在需要的地方嵌套这个组件。
阅读全文
相关推荐
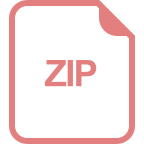
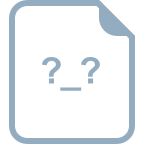
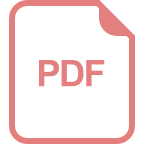













