objective-c实现一个循环队列
时间: 2023-09-03 18:12:21 浏览: 129
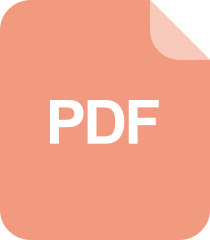
C语言实现循环队列
Objective-C语言中可以使用NSArray或NSMutableArray来实现队列,但是它们是动态数组,插入和删除操作效率较低。如果需要实现一个高效的队列,可以使用循环队列。
循环队列是一种特殊的队列,它的队尾指针可以指向队头位置,形成一个环形结构。这样可以充分利用数组空间,提高队列的效率。
下面是Objective-C实现一个循环队列的示例代码:
```
@interface CircularQueue : NSObject
@property(nonatomic, assign) NSInteger head; // 队头指针
@property(nonatomic, assign) NSInteger tail; // 队尾指针
@property(nonatomic, assign) NSInteger size; // 队列大小
@property(nonatomic, strong) NSMutableArray *queueArray; // 队列数组
- (instancetype)initWithSize:(NSInteger)size; // 初始化方法
- (BOOL)enqueue:(id)obj; // 入队方法
- (id)dequeue; // 出队方法
- (BOOL)isEmpty; // 判断队列是否为空
- (BOOL)isFull; // 判断队列是否已满
@end
@implementation CircularQueue
- (instancetype)initWithSize:(NSInteger)size {
if (self = [super init]) {
self.head = 0;
self.tail = 0;
self.size = size;
self.queueArray = [NSMutableArray arrayWithCapacity:size];
for (NSInteger i = 0; i < size; i++) {
[self.queueArray addObject:[NSNull null]];
}
}
return self;
}
- (BOOL)enqueue:(id)obj {
if ([self isFull]) {
return NO;
}
self.queueArray[self.tail] = obj;
self.tail = (self.tail + 1) % self.size;
return YES;
}
- (id)dequeue {
if ([self isEmpty]) {
return nil;
}
id obj = self.queueArray[self.head];
self.queueArray[self.head] = [NSNull null];
self.head = (self.head + 1) % self.size;
return obj;
}
- (BOOL)isEmpty {
return self.head == self.tail && self.queueArray[self.head] == [NSNull null];
}
- (BOOL)isFull {
return self.head == self.tail && self.queueArray[self.head] != [NSNull null];
}
@end
```
在上面的代码中,我们使用一个NSMutableArray来保存队列元素,使用head和tail两个指针来指示队头和队尾位置。enqueue方法用于入队操作,dequeue方法用于出队操作,isEmpty方法和isFull方法分别用于判断队列是否为空和已满。注意,在enqueue和dequeue方法中,我们使用取模运算来实现循环指针的功能。
使用循环队列可以有效提高队列的效率,特别是在需要频繁插入和删除元素的场景下。
阅读全文
相关推荐
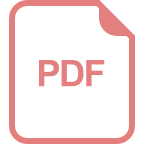

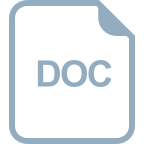
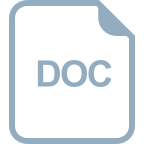

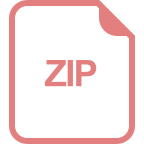
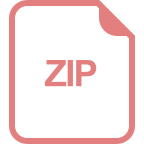
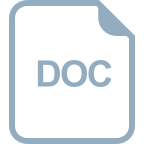
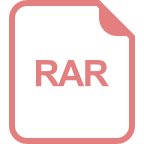
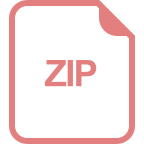
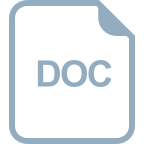

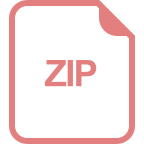
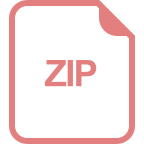
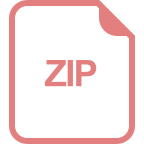
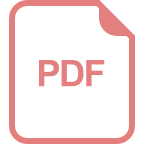
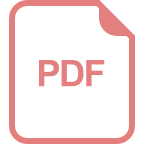