使用objective c实现一个循环队列
时间: 2024-05-04 21:21:47 浏览: 13
Objective-C 中实现循环队列可以使用数组和指针来完成。以下是实现循环队列的示例代码:
```objective-c
@interface CircularQueue : NSObject
@property (nonatomic, assign) NSUInteger capacity; // 队列容量
@property (nonatomic, assign) NSUInteger head; // 队首指针
@property (nonatomic, assign) NSUInteger tail; // 队尾指针
@property (nonatomic, strong) NSMutableArray *items; // 队列元素
- (instancetype)initWithCapacity:(NSUInteger)capacity;
- (BOOL)enqueue:(id)item;
- (id)dequeue;
- (BOOL)isEmpty;
- (BOOL)isFull;
@end
@implementation CircularQueue
- (instancetype)initWithCapacity:(NSUInteger)capacity {
if (self = [super init]) {
_capacity = capacity;
_head = 0;
_tail = 0;
_items = [NSMutableArray arrayWithCapacity:capacity];
}
return self;
}
- (BOOL)enqueue:(id)item {
if ([self isFull]) {
return NO;
}
[self.items addObject:item];
self.tail = (self.tail + 1) % self.capacity;
return YES;
}
- (id)dequeue {
if ([self isEmpty]) {
return nil;
}
id item = self.items[self.head];
self.head = (self.head + 1) % self.capacity;
return item;
}
- (BOOL)isEmpty {
return self.head == self.tail;
}
- (BOOL)isFull {
return (self.tail + 1) % self.capacity == self.head;
}
@end
```
在这个实现中,我们使用了 NSMutableArray 作为存储队列元素的容器,head 和 tail 分别表示队首和队尾的指针,capacity 表示队列容量。enqueue 和 dequeue 方法分别代表入队和出队操作,isEmpty 和 isFull 方法分别用于判断队列是否为空或已满。在 enqueue 操作中,我们使用取模运算来实现循环队列的特性。
相关推荐
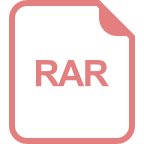
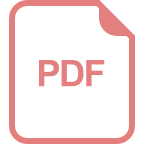














