python re取字符串某一部分
时间: 2023-12-31 08:05:36 浏览: 33
你可以使用正则表达式模块 `re` 中的 `search` 函数来匹配字符串中的某一部分。例如,如果你想从字符串 `"hello world"` 中匹配 `world`,可以使用以下代码:
```python
import re
string = "hello world"
match = re.search(r"\b\w+\b", string)
if match:
print(match.group(0))
```
输出结果为:
```
world
```
在这个例子中,正则表达式 `\b\w+\b` 匹配一个单词,并使用 `search` 函数在字符串中查找匹配项。如果找到了匹配项,`match.group(0)` 就会返回匹配到的字符串。
相关问题
python re 匹配 字符串
在 Python 中,你可以使用 `re` 模块来匹配字符串。这个模块提供了一些函数,可以用正则表达式(regular expression)来匹配和操作字符串。
要使用 `re` 模块,你需要先导入它:
```python
import re
```
然后,你可以使用 `re` 模块中的 `search` 函数来在字符串中查找指定的模式:
```python
import re
string = "Hello, World!"
pattern = "Hello"
match = re.search(pattern, string)
if match:
print("Match found!")
else:
print("Match not found.")
```
这将输出 "Match found!"。
你也可以使用 `findall` 函数来查找字符串中所有符合模式的部分:
```python
import re
string = "Hello, World! How are you doing?"
pattern = r"\b\w+\b"
matches = re.findall(pattern, string)
print(matches)
```
这将输出一个列表,列表中包含了字符串中所有单词:`['Hello', 'World', 'How', 'are', 'you', 'doing']`
正则表达式是一种非常强大的工具,你可以使用它来匹配各种模式,例如数字、日期、电子邮件地址等。有关正则表达式的更多信息,你可以参考 Python 文档中的“正则表达式指南”。
python正则匹配字符串中数字部分
可以使用正则表达式来匹配字符串中的数字部分。例如,匹配字符串中第一个出现的数字可以使用以下代码:
```python
import re
string = "abc123def456"
match = re.search(r'\d+', string)
if match:
print(match.group(0))
```
上述代码中,`re.search()` 函数使用 `\d+` 正则表达式来匹配字符串中的数字部分。`\d` 表示匹配数字,`+` 表示匹配一个或多个数字。如果匹配成功,`match.group(0)` 将返回匹配到的字符串。在本例中,输出结果为 `123`。
如果需要匹配字符串中所有的数字,可以使用 `re.findall()` 函数:
```python
import re
string = "abc123def456"
matches = re.findall(r'\d+', string)
print(matches)
```
上述代码中,`re.findall()` 函数使用 `\d+` 正则表达式来匹配字符串中的数字部分,并返回所有匹配的字符串。在本例中,输出结果为 `['123', '456']`。
相关推荐
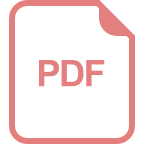
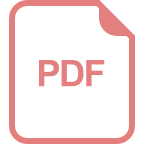
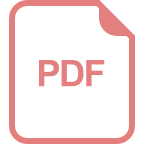












