在一个主窗口中,点击一个链接, 弹出一个子窗口 , 父窗口保留在子窗口中点击关闭, 关闭子窗口. 子窗口的位置位于屏幕的中间
时间: 2023-05-21 13:01:39 浏览: 136
可以使用 JavaScript 来实现这个功能,以下是一个简单的实现代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>弹出子窗口</title>
<script type="text/javascript">
function openChildWindow() {
var childWindow = window.open("child.html", "childWindow", "width=400,height=300,top=" + (screen.height/2-150) + ",left=" + (screen.width/2-200));
childWindow.focus();
}
</script>
</head>
<body>
<a href="#" onclick="openChildWindow(); return false;">点击打开子窗口</a>
</body>
</html>
```
在这个代码中,我们定义了一个 `openChildWindow` 函数,当用户点击链接时,调用这个函数来打开子窗口。子窗口的 URL 是 `child.html`,窗口的名称是 `childWindow`,窗口的大小是 400x300,位置位于屏幕的中间。当用户关闭子窗口时,窗口会自动关闭。
相关问题
通过pyqt5和eric6分别生成了一个主窗口和一个字窗口py文件,使用python通过import两个窗口,实现:在主界面点击按钮后,显示子窗口,并保持子窗口在最前面。当点击子窗口中的某个按钮后,子窗口关闭
在Python中,使用PyQt5库创建GUI应用并集成子窗口通常涉及以下几个步骤:
1. **创建主窗口**(main_window.py):
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
from sub_window import SubWindow
class MainWindow(QMainWindow):
def __init__(self):
super(MainWindow, self).__init__()
self.init_ui()
def init_ui(self):
# 创建一个按钮
button = QPushButton('打开子窗口', self)
button.clicked.connect(self.show_sub_window)
# 设置窗口布局
layout = QVBoxLayout()
layout.addWidget(button)
self.setLayout(layout)
def show_sub_window(self):
sub_window = SubWindow(parent=self) # 创建子窗口实例并指定父窗口为self
sub_window.showMaximized() # 显示最大化,保证其在最前面
sub_window.raise_() # 提升子窗口到顶层
if __name__ == '__main__':
app = QApplication([])
main_win = MainWindow()
main_win.show()
app.exec_()
```
2. **创建子窗口**(sub_window.py):
```python
from PyQt5.QtWidgets import QWidget, QVBoxLayout, QPushButton
class SubWindow(QWidget):
def __init__(self, parent=None):
super(SubWindow, self).__init__(parent)
self.init_ui()
def init_ui(self):
self.setWindowTitle('子窗口')
# 子窗口内另一个按钮
close_button = QPushButton('关闭子窗口', self)
close_button.clicked.connect(self.close_sub_window)
layout = QVBoxLayout()
layout.addWidget(close_button)
self.setLayout(layout)
def close_sub_window(self):
self.close() # 当点击该按钮时,关闭子窗口
# 如果要在主窗口点击按钮后直接显示子窗口,记得在show_sub_window方法里添加创建子窗口的代码
```
当你运行`main_window.py`时,会启动主窗口。在主窗口点击"打开子窗口"按钮后,子窗口将弹出并在最前面显示。子窗口内的"关闭子窗口"按钮用于关闭子窗口。
**相关问题--:**
1. PyQT5中的事件驱动是什么?
2. 如何处理子窗口关闭后的清理工作?
3. 在这个例子中如何避免父子窗口之间的内存泄漏?
给个具体例子,父窗口是一个mainwindow,通过点击按钮显示子窗口dialog,子窗口里面有个按钮,点击可以使用父窗口的funa函数
好的,让我们来看一个具体的例子,假设我们正在使用Qt C++编写一个程序。首先,我们需要创建一个主窗口`MainWindow`,在这个窗口中有一个QPushButton(按钮),点击后会弹出一个`Dialog`子窗口:
```cpp
// MainWindow.h
class MainWindow : public QMainWindow {
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
void showDialog();
private slots:
void on_pushButton_clicked();
};
```
```cpp
// MainWindow.cpp
MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent) {
QPushButton *button = new QPushButton("显示对话框", this);
connect(button, &QPushButton::clicked, this, &MainWindow::showDialog);
// ... 其他窗体设置...
}
void MainWindow::showDialog() {
Dialog dialog(this); // 创建并显示子窗口
dialog.show();
}
```
然后在子窗口`Dialog`中,我们也有一个QPushButton,点击后需要调用`MainWindow`中的`funa()`函数:
```cpp
// Dialog.h
class Dialog : public QDialog {
Q_OBJECT
public:
explicit Dialog(MainWindow *parent = nullptr);
private slots:
void on_pushButton_clicked();
void parentFunctionCall();
private:
MainWindow *parent;
};
```
```cpp
// Dialog.cpp
Dialog::Dialog(MainWindow *parent) : QDialog(parent), parent(parent) {
QPushButton *childButton = new QPushButton("调用父函数", this);
connect(childButton, &QPushButton::clicked, this, &Dialog::parentFunctionCall);
// ... 其他窗口设置...
}
void Dialog::on_pushButton_clicked() {
parent->funa(); // 在这里调用父窗口的funa函数
}
```
当你运行这个程序,点击`MainWindow`的按钮会弹出`Dialog`,再点击`Dialog`的按钮,会执行`parentFunctionCall()`,从而间接调用了`funa()`函数。
阅读全文
相关推荐
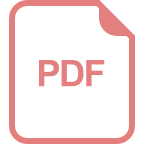
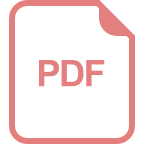


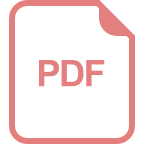
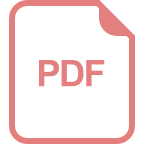
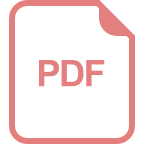
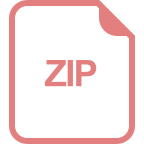
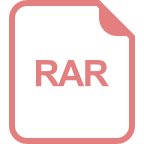
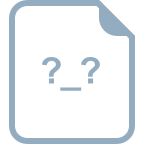
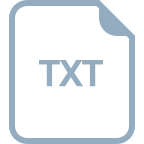
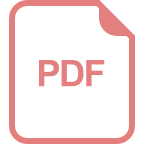
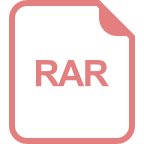