Python编写程序,读取一个存储若干数字的文件,对其中的数字排序后输出
时间: 2024-03-01 11:50:09 浏览: 102
好的,您可以按照以下步骤来实现:
1. 打开文件,读取文件中的数字,并将其存储到一个列表中。
```
with open('filename.txt', 'r') as file:
numbers = [int(num) for num in file.read().split()]
```
2. 对列表中的数字进行排序。
```
numbers.sort()
```
3. 将排序后的数字输出到文件中。
```
with open('sorted_numbers.txt', 'w') as file:
file.write('\n'.join(str(num) for num in numbers))
```
完整的程序代码如下:
```
with open('filename.txt', 'r') as file:
numbers = [int(num) for num in file.read().split()]
numbers.sort()
with open('sorted_numbers.txt', 'w') as file:
file.write('\n'.join(str(num) for num in numbers))
```
其中,`filename.txt` 是存储数字的文件名,`sorted_numbers.txt` 是输出排序后数字的文件名。
相关问题
使用Python编写程序读取一个存储若干数字的文件,对其中的数字排序后输出
您好!感谢您使用ChitGPT。针对您的问题,可以使用Python内置的sort()函数对文件中的数字进行排序。以下是示例代码:
```
with open("filename.txt", "r") as file:
numbers = [int(x) for x in file.readlines()] # 读取文件中的数字
numbers.sort() # 对数字进行排序
print(numbers) # 输出排序后的数字
```
其中,`filename.txt`是存储数字的文件名。首先,我们使用`with...as`语句打开文件,并将文件中的数字读入一个列表中。接着,使用`sort()`函数对列表中的数字进行升序排序。最后,使用`print()`语句输出排序后的数字列表。
希望能解决您的问题,如果您还有其他问题,可以继续提问。
Python编写程序,读取一个储存若干数字的文件,对其中的数字排序后输出
好的,可以使用 Python 的内置函数 `sorted` 来对数字进行排序,具体步骤如下:
1. 打开文件并读取其中的数字,可以使用 `with open()` 来打开文件,然后使用 `readlines()` 来读取其中的内容,并使用 `map` 函数将读取到的字符串转换为数字,最后将其存储到一个列表中。
2. 对列表中的数字进行排序,可以使用 `sorted` 函数来实现,该函数会返回一个新的已排序的列表。
3. 输出排序后的结果,可以使用 `print` 函数来输出已排序的列表。
下面是一个示例代码,可以参考:
```python
with open('numbers.txt', 'r') as f:
numbers = list(map(int, f.readlines()))
sorted_numbers = sorted(numbers)
print(sorted_numbers)
```
其中,`numbers.txt` 是储存数字的文件名,可以根据实际情况进行修改。
阅读全文
相关推荐
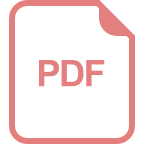












