将一个十进制的正整数 X 转换成 r(r=2~9)进制数,并输出转换后的值。 【实验要求】 要求采用链栈实现,链栈定义如下:
时间: 2024-02-24 08:58:41 浏览: 40
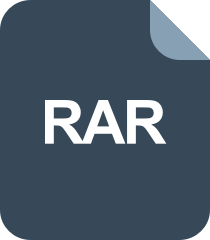
用栈实现进制转换,十进制向其他进制

好的,我能理解您的问题。以下是对您的问题的回答:
链栈的定义如下:
```
typedef struct node {
int data;
struct node* next;
}Node;
typedef struct {
Node* top;
}Stack;
```
其中,`top` 为指向栈顶的指针。接下来,我将给出将一个十进制的正整数 X 转换成 r 进制数的代码实现。请注意,该实现使用链栈来实现。
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node* next;
}Node;
typedef struct {
Node* top;
}Stack;
void init(Stack* s) {
s->top = NULL;
}
int is_empty(Stack* s) {
return s->top == NULL;
}
void push(Stack* s, int x) {
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->data = x;
new_node->next = s->top;
s->top = new_node;
}
int pop(Stack* s) {
if (is_empty(s)) {
printf("Stack is empty!\n");
return -1;
}
int x = s->top->data;
Node* temp = s->top;
s->top = s->top->next;
free(temp);
return x;
}
int main() {
int X, r;
Stack s;
init(&s);
printf("Please enter a positive integer X:\n");
scanf("%d", &X);
printf("Please enter the base r (2 <= r <= 9):\n");
scanf("%d", &r);
while (X != 0) {
push(&s, X % r);
X /= r;
}
printf("The result is:\n");
while (!is_empty(&s)) {
printf("%d", pop(&s));
}
printf("\n");
return 0;
}
```
上述代码实现了将一个十进制的正整数 X 转换成 r 进制数的功能,其中 r 的范围是 2 到 9。感谢您的提问,希望我的回答能够解决您的问题!
阅读全文
相关推荐















