将一个十进制的正整数 X 转换成 r(r=2~9)进制数,并输出转换后的值。要求采用链栈实现,链栈定义如下: typedef struct node { int num; struct node *next; }Lnode,*pNode;
时间: 2024-03-02 21:53:50 浏览: 18
好的,我知道了。以下是代码实现:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int num;
struct node *next;
} Lnode, *pNode;
void push(pNode *top, int num) {
pNode new_node = (pNode) malloc(sizeof(Lnode));
new_node->num = num;
new_node->next = *top;
*top = new_node;
}
int pop(pNode *top) {
if (*top == NULL) {
return -1;
}
int num = (*top)->num;
pNode temp = *top;
*top = temp->next;
free(temp);
return num;
}
int main() {
int x, r;
printf("请输入一个十进制正整数x和要转换的进制r(2~9):\n");
scanf("%d%d", &x, &r);
pNode top = NULL;
while (x != 0) {
push(&top, x % r);
x /= r;
}
printf("转换后的%s进制数为:", r == 2 ? "二" : r == 8 ? "八" : "其它");
while (top != NULL) {
printf("%d", pop(&top));
}
printf("\n");
return 0;
}
```
该程序通过链栈实现了将一个十进制的正整数 X 转换成 r(r=2~9)进制数,并输出转换后的值。其中,push()和pop()函数实现链栈的入栈和出栈操作。在主函数中,先输入要转换的十进制数和进制,然后将十进制数不断除以进制取余,并将余数压入栈中,最后依次出栈输出。
相关推荐
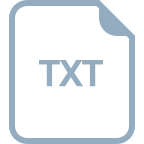














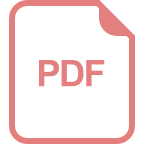