def move_towards_player2(self, player): # Find direction vector (dx, dy) between enemy and player. if pygame.math.Vector2(player.rect.x - self.rect.x,player.rect.y - self.rect.y)==(): dirvect=pygame.math.Vector2(10,10) else: dirvect = pygame.math.Vector2(player.rect.x - self.rect.x, player.rect.y - self.rect.y) dirvect.normalize() # Move along this normalized vector towards the player at current speed. dirvect.scale_to_length(self.speed) self.rect.move_ip(dirvect)
时间: 2023-06-16 17:04:25 浏览: 187
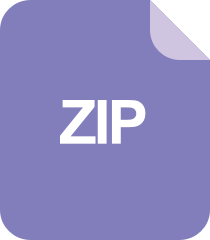
Java-IEEE-2012-basepapers-List-2.zip_site:www.pudn.com
这是一个 Python 代码片段,看起来是一个敌人向玩家移动的函数。首先,它计算了敌人和玩家之间的方向向量(dx,dy),然后将其标准化。标准化后,它根据当前速度将其缩放到所需的长度,并使用 `move_ip` 方法移动敌人的矩形。
这个函数使用了 Pygame 向量模块中的 `Vector2` 类,它提供了向量相关的操作和方法,包括向量的计算、标准化、缩放等。`normalize` 方法将向量标准化,使其长度为1,这意味着它的方向不变,但长度为1,方便后续缩放操作。`scale_to_length` 方法将向量缩放到指定的长度,这里使用了敌人的速度作为长度。最后,`move_ip` 方法将敌人的矩形沿着方向向量移动。
阅读全文
相关推荐
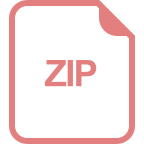
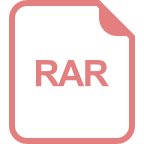


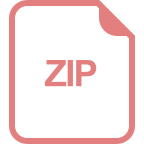
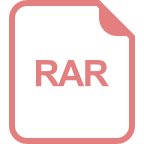
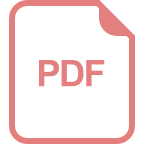
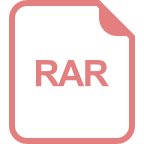
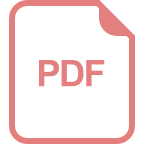
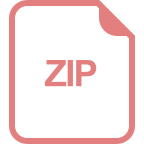
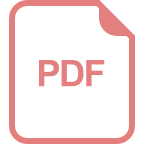
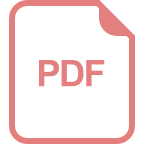
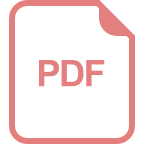




