c字符串数组字典序排序
时间: 2023-08-23 17:30:36 浏览: 150
你可以使用 C++ 中的 std::sort 函数来对字符串数组进行字典序排序。下面是一个示例代码:
```c++
#include <iostream>
#include <algorithm>
#include <cstring>
using namespace std;
const int MAXN = 1005; // 最大字符串数
const int MAXM = 105; // 最大字符串长度
char str[MAXN][MAXM]; // 存储字符串的数组
bool cmp(char *a, char *b) {
return strcmp(a, b) < 0; // 按照字典序排序
}
int main() {
int n; // 字符串个数
cin >> n;
for (int i = 0; i < n; i++) {
cin >> str[i];
}
sort(str, str + n, cmp); // 使用 sort 函数进行排序
for (int i = 0; i < n; i++) {
cout << str[i] << endl;
}
return 0;
}
```
这段代码首先输入字符串个数和每个字符串,然后使用 `sort` 函数进行排序,最后输出排序后的字符串数组。其中,`cmp` 函数是用来比较两个字符串的大小关系,如果第一个字符串小于第二个字符串,则返回 true。
相关问题
c语言字符串数组字典排序
C语言中,字符串数组字典排序是指按照字典序对字符串数组进行排序。字典序是指按照字母表顺序进行比较的方法。例如,"apple"在字典序上比"banana"小,因为"a"在字母表中比"b"小。
下面是一种实现字符串数组字典排序的方法:
1. 定义一个字符串数组,存储需要排序的字符串。
2. 使用冒泡排序或选择排序等算法对字符串数组进行排序。
3. 在排序过程中,比较两个字符串的字典序大小,如果需要交换位置,则交换两个字符串在数组中的位置。
下面是一个示例代码:
```
#include <stdio.h>
#include <string.h>
void sort(char arr[][100], int n) {
int i, j;
char temp[100];
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (strcmp(arr[j], arr[j + 1]) > 0) {
strcpy(temp, arr[j]);
strcpy(arr[j], arr[j + 1]);
strcpy(arr[j + 1], temp);
}
}
}
}
int main() {
char arr[5][100];
int i;
printf("请输入5个字符串:\n");
for (i = 0; i < 5; i++) {
scanf("%s", arr[i]);
}
sort(arr, 5);
printf("排序后的字符串数组为:\n");
for (i = 0; i < 5; i++) {
printf("%s\n", arr[i]);
}
return 0;
}
```
C语言怎么对字符串进行字典序排序
在C语言中,对字符串进行字典序排序通常可以使用标准库函数`qsort()`结合`strcmp()`函数。这里是一个基本的示例,假设我们有一个字符指针数组`strings[]`和一个整数数组`lengths[]`,分别表示字符串和它们的长度,我们可以这样做:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义比较函数,用于字符串字典序排序
int compare_strings(const void *a, const void *b) {
const char **strA = (const char **)a;
const char **strB = (const char **)b;
return strcmp(*strA, *strB); // 字符串直接通过strcmp比较
}
int main() {
int n;
printf("Enter the number of strings: ");
scanf("%d", &n);
// 假设strings和lengths已初始化,存储了字符串及其长度
char **strings = malloc(n * sizeof(char *));
int *lengths = malloc(n * sizeof(int));
// ... (填充字符串和长度)
qsort(strings, n, sizeof(char*), compare_strings); // 使用qsort对字符串进行排序
for (int i = 0; i < n; i++) {
printf("%s\n", strings[i]); // 输出排序后的字符串
}
free(strings);
free(lengths);
return 0;
}
```
在这个例子中,`compare_strings()`函数作为`qsort()`的第三个参数,它告诉`qsort()`如何比较两个元素(即字符串)。`strcmp()`返回值是负数、零或正数,分别表示第一个字符串在字典顺序上位于第二个字符串之前、相等或之后。
阅读全文
相关推荐
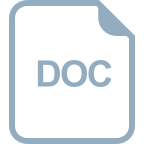
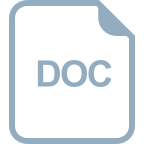

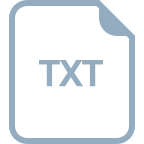
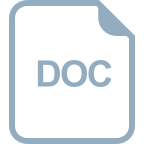
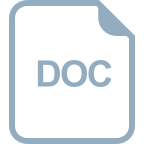







