C语言中qsort函数的使用方法及示例
时间: 2023-05-17 14:02:40 浏览: 84
qsort函数是C语言中的一个标准库函数,用于对数组进行快速排序。它的使用方法如下:
void qsort(void *base, size_t nmemb, size_t size, int (*compar)(const void *, const void *));
其中,base是要排序的数组的首地址,nmemb是数组中元素的个数,size是每个元素的大小,compar是一个函数指针,用于比较两个元素的大小关系。
下面是一个示例代码,用于对一个整型数组进行升序排序:
#include <stdio.h>
#include <stdlib.h>
int compare(const void *a, const void *b) {
return (*(int*)a - *(int*)b);
}
int main() {
int arr[] = {5, 3, 8, 4, 2};
int n = sizeof(arr) / sizeof(arr[0]);
qsort(arr, n, sizeof(int), compare);
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
return 0;
}
输出结果为:2 3 4 5 8
注意:在使用qsort函数时,需要自己编写比较函数,以指定排序的方式。在上面的示例代码中,compare函数用于升序排序。
相关问题
自己使用c语言实现qsort函数
qsort函数是C标准库中的一个快速排序函数,用于对数组进行排序。下面是一个简单的示例,展示如何使用C语言实现qsort函数:
```c
#include <stdio.h>
// 比较函数,用于指定排序规则
int compare(const void *a, const void *b) {
// 转换为指向int类型的指针
int *x = (int *)a;
int *y = (int *)b;
if (*x < *y) {
return -1;
} else if (*x > *y) {
return 1;
} else {
return 0;
}
}
// 快速排序函数
void quicksort(int arr[], int low, int high) {
if (low < high) {
int pivot = arr[low];
int i = low + 1;
int j = high;
while (i <= j) {
while (i <= j && arr[i] <= pivot) {
i++;
}
while (i <= j && arr[j] > pivot) {
j--;
}
if (i < j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[low];
arr[low] = arr[j];
arr[j] = temp;
quicksort(arr, low, j - 1);
quicksort(arr, j + 1, high);
}
}
int main() {
int arr[] = {5, 2, 8, 9, 1};
int n = sizeof(arr) / sizeof(arr[0]);
// 使用自定义的比较函数进行排序
qsort(arr, n, sizeof(int), compare);
printf("Sorted array: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
```
在这个示例中,我们定义了一个compare函数作为qsort函数的比较函数。这个比较函数接收两个指针参数,指向要比较的元素。函数内部将这些指针转换为int类型的指针,并根据需要的排序规则返回-1、0或1。
然后,我们使用自定义的quicksort函数来实现快速排序。这个函数使用了经典的快速排序算法来对数组进行排序。
最后,在main函数中,我们声明一个整型数组arr,并使用qsort函数对其进行排序。排序完成后,我们打印出排序后的数组。
请注意,这只是一个简单的示例,用于演示如何使用C语言实现一个类似于qsort函数的功能。在实际使用中,建议使用C标准库提供的qsort函数,因为它经过了优化,并且适用于各种数据类型。
C语言使用qsort函数对链表排序
C语言的qsort函数只能对数组进行排序,对于链表的排序可以使用其他算法,比如插入排序或归并排序。
以下是使用插入排序对链表进行排序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int value;
struct Node* next;
} Node;
void insert(Node** head, int value) {
Node* new_node = malloc(sizeof(Node));
new_node->value = value;
new_node->next = *head;
*head = new_node;
}
void print_list(Node* head) {
while (head != NULL) {
printf("%d ", head->value);
head = head->next;
}
printf("\n");
}
void insertion_sort(Node** head) {
Node* sorted_list = NULL;
Node* current = *head;
while (current != NULL) {
Node* next = current->next;
if (sorted_list == NULL || current->value < sorted_list->value) {
current->next = sorted_list;
sorted_list = current;
} else {
Node* temp = sorted_list;
while (temp->next != NULL && temp->next->value < current->value) {
temp = temp->next;
}
current->next = temp->next;
temp->next = current;
}
current = next;
}
*head = sorted_list;
}
int main() {
Node* head = NULL;
insert(&head, 5);
insert(&head, 2);
insert(&head, 8);
insert(&head, 1);
insert(&head, 4);
printf("Before sorting: ");
print_list(head);
insertion_sort(&head);
printf("After sorting: ");
print_list(head);
return 0;
}
```
输出结果为:
```
Before sorting: 4 1 8 2 5
After sorting: 1 2 4 5 8
```
相关推荐
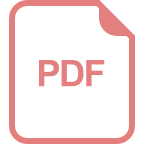
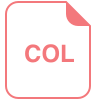
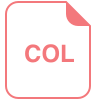










