c语言qsort函数排序结构体
时间: 2024-09-14 22:17:22 浏览: 51
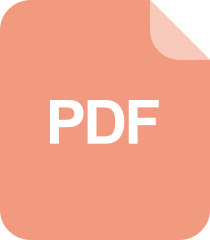
C语言中qsort函数用法实例小结
`qsort` 函数是 C 语言标准库中的一个通用排序函数,它可以用来对数组进行排序。当需要对结构体数组进行排序时,可以通过传递一个比较函数来指定排序的依据。比较函数是用户自定义的,它按照结构体中的某个字段的值来进行比较。
下面是一个使用 `qsort` 对结构体数组进行排序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义一个结构体
typedef struct {
int id;
char name[50];
} Student;
// 比较函数,根据结构体中的 id 字段进行比较
int compare_by_id(const void *a, const void *b) {
const Student *studentA = (const Student *)a;
const Student *studentB = (const Student *)b;
return studentA->id - studentB->id;
}
int main() {
// 创建并初始化一个结构体数组
Student students[] = {
{3, "Alice"},
{1, "Bob"},
{2, "Charlie"}
};
int array_size = sizeof(students) / sizeof(students[0]);
// 使用 qsort 和比较函数进行排序
qsort(students, array_size, sizeof(Student), compare_by_id);
// 打印排序后的结果
for(int i = 0; i < array_size; i++) {
printf("ID: %d, Name: %s\n", students[i].id, students[i].name);
}
return 0;
}
```
在这个示例中,`compare_by_id` 函数按照结构体中的 `id` 字段来比较两个 `Student` 结构体的大小。然后使用 `qsort` 函数对 `students` 数组进行排序,其中指定了数组的长度、每个元素的大小以及比较函数。
使用 `qsort` 对结构体数组进行排序时,需要特别注意比较函数的编写和元素大小的正确传递,这样才能保证排序的正确性。
阅读全文
相关推荐
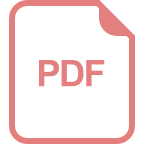
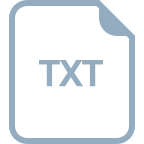



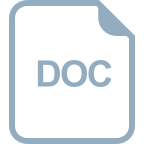
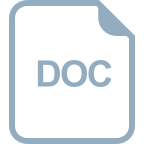
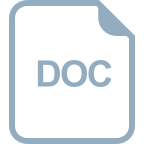
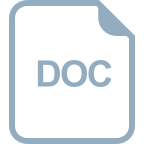
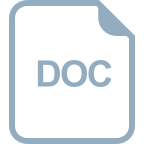
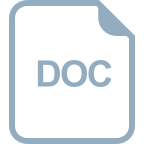






