编写代码实现一个图灵机,输出字符串”hello,world”后停机
时间: 2024-03-15 20:47:43 浏览: 65
下面是一个Python实现的图灵机,可以输出字符串"hello, world"后停机:
```python
class TuringMachine:
def __init__(self, program, tape):
self.program = program
self.tape = tape
self.head = 0
self.state = program.initial_state
def step(self):
symbol = self.tape[self.head]
if symbol in self.program.rules[self.state]:
new_state, new_symbol, direction = self.program.rules[self.state][symbol]
self.tape[self.head] = new_symbol
self.state = new_state
self.head += direction
return True
else:
return False
def run(self):
while self.step():
pass
class Program:
def __init__(self, rules, initial_state):
self.rules = rules
self.initial_state = initial_state
tape = list("________________")
tape[0] = "h"
tape[1] = "e"
tape[2] = "l"
tape[3] = "l"
tape[4] = "o"
tape[5] = ","
tape[6] = " "
tape[7] = "w"
tape[8] = "o"
tape[9] = "r"
tape[10] = "l"
tape[11] = "d"
tape[12] = "_"
rules = {
"q0": {
"h": ("q1", "h", 1),
"_": ("qf", "_", 0)
},
"q1": {
"e": ("q2", "e", 1)
},
"q2": {
"l": ("q3", "l", 1)
},
"q3": {
"l": ("q4", "l", 1)
},
"q4": {
"o": ("q5", "o", 1)
},
"q5": {
",": ("q6", ",", 1)
},
"q6": {
" ": ("q7", " ", 1)
},
"q7": {
"w": ("q8", "w", 1)
},
"q8": {
"o": ("q9", "o", 1)
},
"q9": {
"r": ("q10", "r", 1)
},
"q10": {
"l": ("q11", "l", 1)
},
"q11": {
"d": ("qf", "d", 1)
}
}
program = Program(rules, "q0")
tm = TuringMachine(program, tape)
tm.run()
print("".join(tm.tape))
```
这个图灵机使用一个列表来模拟纸带,初始状态下纸带上的所有符号都为"_",即空格。每个状态都对应一个字典,键为当前读头所在的符号,值为一个三元组,分别表示转移到的新状态、要写入的新符号,以及读头移动的方向。在运行时,图灵机不断读取当前符号,根据当前状态和规则进行状态转移和符号修改,最终输出"hello, world"并停机。
阅读全文
相关推荐
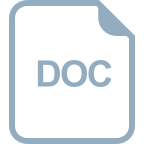
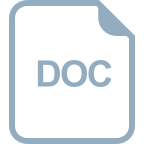
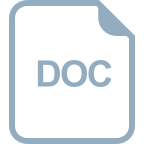


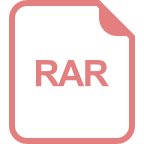
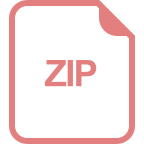
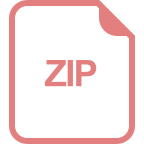
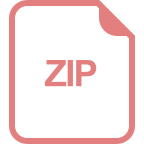
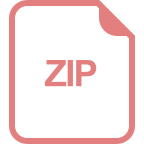
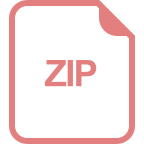
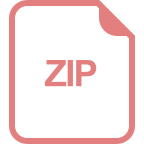
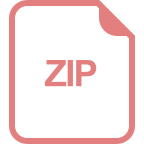
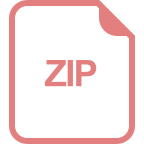
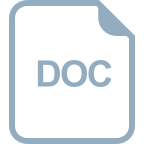
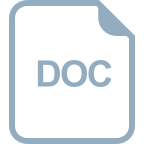
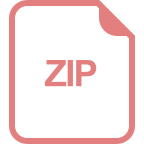
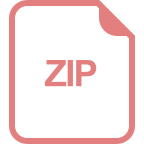
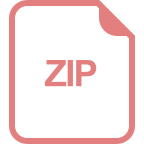