react 路由跳转 固定携带一个参数
时间: 2023-11-17 17:06:14 浏览: 35
可以使用 React Router 的 `Link` 组件来进行路由跳转,并在 `to` 属性中指定要跳转的路径和参数。
例如,如果要跳转到 `/user` 页面,并携带一个名为 `userId` 的参数,可以这样写:
```jsx
import { Link } from 'react-router-dom';
<Link to={{ pathname: '/user', search: '?userId=123' }}>Go to User</Link>
```
在上面的代码中,`pathname` 属性表示跳转的路径,`search` 属性表示要携带的参数。在这里,参数为 `userId=123`。
在接收参数的组件中,可以通过 `props.location.search` 获取到参数字符串,然后使用 URLSearchParams API 解析参数值。
例如,在 `/user` 组件中,可以这样获取并解析参数:
```jsx
import { useLocation } from 'react-router-dom';
function User() {
const location = useLocation();
const searchParams = new URLSearchParams(location.search);
const userId = searchParams.get('userId');
// ...
}
```
这样就可以获取到跳转时携带的 `userId` 参数了。
相关问题
react 路由跳转固定携带一个参数
React 路由可以通过 URL 中的参数进行传递,例如:`/user/123`,其中 `123` 就是参数。如果想要固定携带一个参数,可以在路由配置中设置默认参数。假设要跳转到 `/user` 页面,并且固定携带参数 `id=123`,可以这样配置路由:
```jsx
import { BrowserRouter as Router, Switch, Route, Link, useParams } from 'react-router-dom';
function App() {
return (
<Router>
<nav>
<ul>
<li>
<Link to="/user">User</Link>
</li>
</ul>
</nav>
<Switch>
<Route path="/user/:id?" component={User} />
</Switch>
</Router>
);
}
function User() {
const { id } = useParams();
return <h1>User {id || '123'}</h1>;
}
```
在上面的代码中,`Route` 组件的 `path` 属性设置为 `/user/:id?`,其中 `:id?` 表示参数 `id` 是可选的。在 `User` 组件中,通过 `useParams` 钩子可以获取到 `id` 参数。如果 URL 中没有传递 `id` 参数,则会使用默认值 `123`。这样就可以固定携带一个参数进行路由跳转了,例如:`<Link to="/user">User</Link>`。
react路由跳转携带参数
在React路由中,可以使用React Router提供的withRouter高阶组件或者Hook来获取当前路由的参数。
使用withRouter:
```javascript
import { withRouter } from 'react-router-dom';
class MyComponent extends React.Component {
handleClick = () => {
const { history } = this.props;
history.push(`/path/${paramValue}`);
}
render() {
return (
<button onClick={this.handleClick}>跳转</button>
);
}
}
export default withRouter(MyComponent);
```
使用Hook:
```javascript
import { useHistory } from 'react-router-dom';
function MyComponent() {
const history = useHistory();
const handleClick = () => {
history.push(`/path/${paramValue}`);
}
return (
<button onClick={handleClick}>跳转</button>
);
}
export default MyComponent;
```
在路由配置中,可以使用动态路由来获取参数:
```javascript
<Route path="/path/:paramValue" component={MyComponent} />
```
在MyComponent组件中,可以使用props.match.params.paramValue来获取参数值。例如:
```javascript
import { withRouter } from 'react-router-dom';
class MyComponent extends React.Component {
handleClick = () => {
const { history, match } = this.props;
history.push(`/path/${match.params.paramValue}`);
}
render() {
const { match } = this.props;
const paramValue = match.params.paramValue;
return (
<div>{paramValue}</div>
);
}
}
export default withRouter(MyComponent);
```
相关推荐
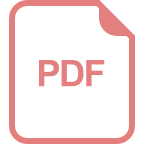
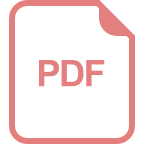
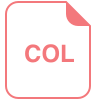
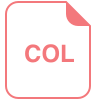
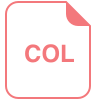
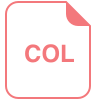








