力扣317python
时间: 2023-10-01 10:02:32 浏览: 121
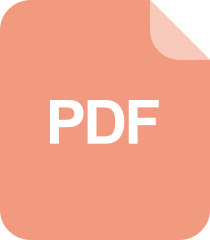
力扣
力扣317题的Python解法如下:
```python
from collections import deque
def shortestDistance(grid):
if not grid or not grid[0]:
return -1
rows, cols = len(grid), len(grid[0])
buildings = 0
distance = [[0] * cols for _ in range(rows)]
reach = [[0] * cols for _ in range(rows)]
for i in range(rows):
for j in range(cols):
if grid[i][j] == 1:
buildings += 1
if not bfs(grid, distance, reach, i, j):
return -1
min_distance = float('inf')
for i in range(rows):
for j in range(cols):
if grid[i][j] == 0 and reach[i][j] == buildings:
min_distance = min(min_distance, distance[i][j])
return min_distance if min_distance != float('inf') else -1
def bfs(grid, distance, reach, row, col):
rows, cols = len(grid), len(grid[0])
visited = [[False] * cols for _ in range(rows)]
queue = deque([(row, col)])
visited[row][col] = True
level = 0
while queue:
level += 1
size = len(queue)
for _ in range(size):
x, y = queue.popleft()
neighbors = [(x-1, y), (x+1, y), (x, y-1), (x, y+1)]
for nx, ny in neighbors:
if 0 <= nx < rows and 0 <= ny < cols and not visited[nx][ny]:
visited[nx][ny] = True
if grid[nx][ny] == 0:
distance[nx][ny] += level
reach[nx][ny] += 1
queue.append((nx, ny))
elif grid[nx][ny] == 1:
continue
else:
return False # obstacle
return True
```
这段代码用于解决力扣317题,是一个基于广度优先搜索的解法。它计算了每个空地到所有建筑物的最短距离,并找到距离所有建筑物最近的空地。如果有多个最近的空地,则返回其中任意一个的距离。如果不存在这样的空地,则返回-1。
阅读全文
相关推荐
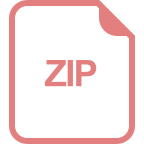
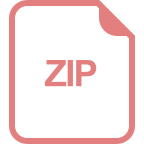
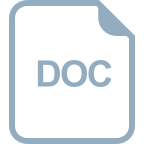














