独立编写一个程序,实现字符串模式匹配 BF 算法。在Main函数中调用自己实现BF算法的函数(使用c语言)
时间: 2024-10-11 11:04:30 浏览: 19
在C语言中,我们可以使用Breadth First Search (BF) 或者更常见的 Knuth-Pratt (KMP) 算法来实现字符串模式匹配。这里我们先讲解一下Brute Force(穷举搜索)的简单版本,它虽然效率较低,但在教学和理解原理时是一个很好的起点。
```c
#include <stdio.h>
#include <string.h>
// 功能:BF搜索字符串str中是否包含模式pat
int bf_search(char* str, char* pat) {
int lenStr = strlen(str);
int lenPat = strlen(pat);
// 搜索过程
for (int i = 0; i <= lenStr - lenPat; i++) {
int j;
for (j = 0; j < lenPat; j++) {
if (str[i + j] != pat[j]) {
if (j == 0) { // 如果刚刚开始比较,当前字符不匹配,跳到下一个位置继续
i++;
continue;
} else {
break; // 否则直接跳出循环
}
}
}
// 如果整个模式都匹配了,返回匹配的位置
if (j == lenPat)
return i;
}
// 如果没有找到匹配,返回-1
return -1;
}
int main() {
char str[] = "Hello, World!";
char pat[] = "World";
int result = bf_search(str, pat);
if (result != -1)
printf("Pattern found at position %d\n", result);
else
printf("Pattern not found in the string.\n");
return 0;
}
```
这个程序在`main`函数中调用了`bf_search`函数,该函数通过逐个字符比较的方式查找模式在主串中是否存在。注意,Brute Force搜索的时间复杂度是O(nm),其中n是主串长度,m是模式长度,对于大规模数据可能会很慢。
阅读全文
相关推荐









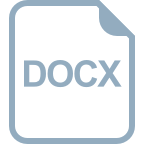
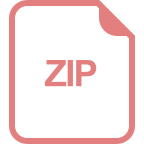
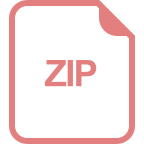
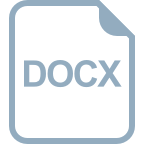
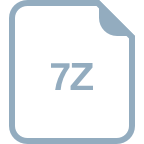
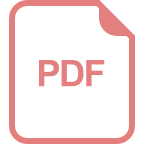