std::string find的用法
时间: 2023-11-20 07:05:15 浏览: 170
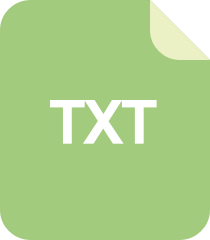
find的用法很有用
std::string的find函数用于在字符串中查找指定子字符串的位置。如果找到了该子字符串,则返回该子字符串在原字符串中的位置;如果没有找到,则返回std::string::npos。该函数有多个重载版本,可以指定查找的起始位置和查找的字符个数等参数。下面是两个使用find函数的例子:
```
//例子1
std::string str("abcdefg");
std::string::size_type pos = str.find("abc");
if (pos != std::string::npos) {
cout << "找到了,位置是:" << pos << endl;
} else {
cout << "没找到" << endl;
}
//例子2
std::string str("abcdefg");
if (str.find("abc") != std::string::npos) {
cout << "找到了" << endl;
} else {
cout << "没找到" << endl;
}
```
阅读全文
相关推荐
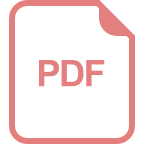







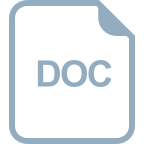
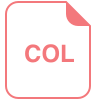
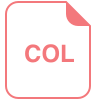





