ESP8266WebServer server(80);esp01s作为wed服务器实时接收arduino r3用软串口发送的json型数据mySerial.println(jsonString);的代码详细化
时间: 2023-06-16 11:05:16 浏览: 153
以下是ESP8266WebServer的基本使用示例,可以实现在ESP8266上创建Web服务器并接收HTTP请求:
```
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
ESP8266WebServer server(80);
void handleRoot() {
server.send(200, "text/plain", "Hello World!");
}
void setup() {
WiFi.begin("SSID", "password"); // 连接Wi-Fi网络
while (WiFi.status() != WL_CONNECTED) { // 等待连接成功
delay(1000);
}
server.on("/", handleRoot); // 注册处理函数
server.begin(); // 启动Web服务器
}
void loop() {
server.handleClient(); // 监听HTTP请求
}
```
如果要实时接收Arduino发送的JSON数据,可以在`handleRoot()`函数中解析接收到的数据并进行处理。假设Arduino发送的JSON数据格式如下:
```
{
"sensor": "temperature",
"value": 25.0
}
```
可以使用ArduinoJson库来解析JSON数据,示例代码如下:
```
#include <ArduinoJson.h>
void handleRoot() {
if (server.method() == HTTP_POST) { // 判断HTTP请求方法是否为POST
StaticJsonDocument<128> doc; // 创建JSON文档
DeserializationError error = deserializeJson(doc, server.arg("plain")); // 解析JSON数据
if (error) {
server.send(400, "text/plain", "Bad Request"); // 解析失败,返回错误码
} else {
const char* sensor = doc["sensor"]; // 获取传感器类型
float value = doc["value"]; // 获取传感器数值
// 处理数据...
server.send(200, "text/plain", "OK"); // 处理成功,返回OK
}
} else {
server.send(405, "text/plain", "Method Not Allowed"); // 非POST请求,返回错误码
}
}
```
在Arduino中使用软串口发送JSON数据的代码可能类似于以下内容:
```
#include <SoftwareSerial.h>
#include <ArduinoJson.h>
SoftwareSerial mySerial(2, 3); // 初始化软串口
void setup() {
mySerial.begin(9600); // 启动软串口
}
void loop() {
StaticJsonDocument<128> doc; // 创建JSON文档
doc["sensor"] = "temperature"; // 设置传感器类型
doc["value"] = 25.0; // 设置传感器数值
char jsonString[128]; // 创建JSON字符串缓冲区
serializeJson(doc, jsonString, sizeof(jsonString)); // 将JSON数据序列化为字符串
mySerial.println(jsonString); // 发送JSON字符串
delay(1000); // 等待1秒
}
```
需要将软串口接收到的JSON数据转发到ESP8266上,可以使用Serial接收数据并通过WiFiClient发送HTTP请求的方式实现。可以在ESP8266WebServer的回调函数中添加以下代码:
```
void handleSerial() {
if (mySerial.available() > 0) {
String jsonString = mySerial.readStringUntil('\n'); // 读取一行JSON字符串
WiFiClient client; // 创建WiFiClient
if (client.connect("192.168.1.100", 80)) { // 连接ESP8266的IP地址和端口号
client.println("POST / HTTP/1.1"); // 发送HTTP请求
client.println("Content-Type: application/json");
client.print("Content-Length: ");
client.println(jsonString.length());
client.println();
client.println(jsonString); // 发送JSON数据
client.stop(); // 断开连接
}
}
}
```
最后在`loop()`函数中调用`handleSerial()`函数即可。注意,在使用WiFiClient发送HTTP请求时,需要提前将ESP8266连接到Wi-Fi网络。
阅读全文
相关推荐















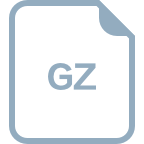