可以帮我用c++写图的广度优先遍历程序吗
时间: 2023-08-31 08:21:18 浏览: 161
### 回答1:
当然可以!我可以帮助您编写使用 C 语言实现图的广度优先遍历的程序。
下面是一个示例程序,该程序使用邻接矩阵存储图并实现了广度优先遍历。
```
#include <stdio.h>
#include <stdbool.h>
#define MAX_VERTICES 100
int graph[MAX_VERTICES][MAX_VERTICES]; // 图的邻接矩阵
int num_vertices; // 图中顶点的数量
bool visited[MAX_VERTICES]; // 记录每个顶点是否已被访问
// 队列的数据结构
typedef struct {
int queue[MAX_VERTICES];
int front;
int rear;
} Queue;
// 初始化队列
void init_queue(Queue *q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
bool queue_is_empty(Queue *q) {
return q->front == q->rear;
}
// 入队
void enqueue(Queue *q, int v) {
q->queue[q->rear++] = v;
}
// 出队
int dequeue(Queue *q) {
return q->queue[q->front++];
}
// 广度优先遍历
void bfs(int start) {
Queue q;
init_queue(&q);
// 将起点加入队列并标记为已访问
enqueue(&q, start);
visited[start] = true;
while (!queue_is_empty(&q)) {
int v = dequeue(&q); // 取出队头元素
printf("%d ", v); // 访问该顶点
// 找到与 v 相邻且未被访问的顶点,并加入队列
for (int i = 0; i < num_vertices; i++) {
if (graph[v][i] != 0 && !visited[i]) {
enqueue(&q, i);
visited[i] = true;
}
}
}
}
int main(void) {
### 回答2:
当然可以!下面是一个用C语言实现图的广度优先遍历的程序:
```c
#include<stdio.h>
#include<stdlib.h>
#define MAX_SIZE 100
// 邻接表中的顶点节点
struct vertexNode {
int data; // 顶点数据
struct vertexNode* next; // 指向下一个顶点节点的指针
};
// 图的邻接表
struct graph {
int numVertices; // 图中顶点的数量
struct vertexNode** adjLists; // 指向邻接表的指针数组
int* visited; // 记录顶点是否被访问过的数组
};
// 创建新的顶点节点
struct vertexNode* createVertexNode(int data) {
struct vertexNode* newNode = malloc(sizeof(struct vertexNode));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 创建图
struct graph* createGraph(int numVertices) {
struct graph* newGraph = malloc(sizeof(struct graph));
newGraph->numVertices = numVertices;
// 创建邻接表的指针数组
newGraph->adjLists = malloc(numVertices * sizeof(struct vertexNode*));
// 创建顶点访问标志的数组
newGraph->visited = malloc(numVertices * sizeof(int));
int i;
for(i = 0; i < numVertices; i++) {
newGraph->adjLists[i] = NULL;
newGraph->visited[i] = 0;
}
return newGraph;
}
// 添加边到有向图中
void addEdge(struct graph* graph, int src, int dest) {
// 添加边从src到dest的情况
struct vertexNode* newNode = createVertexNode(dest);
newNode->next = graph->adjLists[src];
graph->adjLists[src] = newNode;
// 添加边从dest到src的情况(因为是无向图)
newNode = createVertexNode(src);
newNode->next = graph->adjLists[dest];
graph->adjLists[dest] = newNode;
}
// 广度优先遍历
void breadthFirstTraversal(struct graph* graph, int startVertex) {
int queue[MAX_SIZE]; // 创建一个循环队列
int front = -1, rear = -1; // 初始时队首和队尾都为-1
// 将起始顶点标记为已访问
graph->visited[startVertex] = 1;
queue[++rear] = startVertex; // 将起始顶点入队
printf("广度优先遍历结果:");
while(front != rear) { // 当队列不为空时
int currentVertex = queue[++front]; // 队首元素出队
printf("%d ", currentVertex);
struct vertexNode* temp = graph->adjLists[currentVertex];
// 遍历与当前顶点相连的所有顶点
while(temp) {
int adjVertex = temp->data;
// 如果该顶点尚未被访问,则将其标记为已访问并入队
if(graph->visited[adjVertex] == 0) {
graph->visited[adjVertex] = 1;
queue[++rear] = adjVertex;
}
temp = temp->next;
}
}
}
// 主函数
int main() {
int numVertices, numEdges;
printf("请输入顶点的数量和边的数量:");
scanf("%d %d", &numVertices, &numEdges);
struct graph* graph = createGraph(numVertices);
printf("请输入每条边的起始顶点和结束顶点:\n");
int i, src, dest;
for(i = 0; i < numEdges; i++) {
scanf("%d %d", &src, &dest);
addEdge(graph, src, dest);
}
int startVertex;
printf("请输入起始顶点:");
scanf("%d", &startVertex);
breadthFirstTraversal(graph, startVertex);
printf("\n");
return 0;
}
```
希望以上程序能够满足您的需求!
### 回答3:
当然可以帮您编写使用 C 语言实现图的广度优先遍历程序。
首先,我们需要定义一个图的数据结构来表示图。可以使用邻接矩阵或邻接表来存储图的信息。这里我们选择使用邻接矩阵。
```
#define MAX_NUM_VERTICES 100
typedef struct {
int vertices[MAX_NUM_VERTICES]; // 顶点数组
int edges[MAX_NUM_VERTICES][MAX_NUM_VERTICES]; // 邻接矩阵
int numVertices; // 图中的顶点数
} Graph;
```
接下来,我们定义一个队列数据结构来辅助广度优先遍历算法。队列用于存储待访问的顶点。
```
#define MAX_QUEUE_SIZE 100
typedef struct {
int data[MAX_QUEUE_SIZE];
int front; // 队列头部索引
int rear; // 队列尾部索引
} Queue;
```
然后,我们可以实现广度优先遍历算法。该算法从指定起始顶点开始,逐层访问其邻接顶点,直到遍历完整个图。
```
#include <stdio.h>
void BFS(Graph* graph, int startVertex) {
int visited[MAX_NUM_VERTICES] = {0}; // 记录顶点是否已被访问
Queue queue;
int i, currentVertex;
queue.front = queue.rear = -1;
enqueue(&queue, startVertex); // 将起始顶点入队
visited[startVertex] = 1; // 标记起始顶点已访问
while (!isEmpty(&queue)) {
currentVertex = dequeue(&queue); // 出队
printf("%d ", currentVertex); // 访问顶点
for (i = 0; i < graph->numVertices; i++) {
if (graph->edges[currentVertex][i] && !visited[i]) {
enqueue(&queue, i); // 将未访问的邻接顶点入队
visited[i] = 1; // 标记已访问
}
}
}
}
void enqueue(Queue* queue, int data) {
queue->rear++;
queue->data[queue->rear] = data;
}
int dequeue(Queue* queue) {
return queue->data[++queue->front];
}
int isEmpty(Queue* queue) {
return queue->front == queue->rear;
}
```
最后,调用 BFS 函数并传入图和起始顶点的索引即可完成广度优先遍历。
```
Graph graph;
// 初始化图,设置顶点和边的信息
BFS(&graph, startVertex);
```
以上就是使用 C 语言实现图的广度优先遍历的程序。希望对您有帮助!
阅读全文
相关推荐



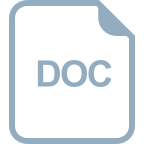
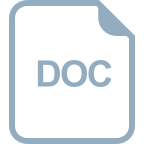









