C#如何用GDI画一个圆角矩形
时间: 2023-07-27 17:12:52 浏览: 182
可以使用 `GraphicsPath` 类来绘制圆角矩形,具体步骤如下:
1. 创建一个 `GraphicsPath` 对象。
2. 使用 `AddArc` 方法添加矩形的四个角的弧线。
3. 使用 `AddLine` 方法在弧线之间绘制直线段。
4. 使用 `CloseFigure` 方法关闭路径。
5. 使用 `DrawPath` 方法将路径绘制到画布上。
下面是一个示例代码:
```csharp
private void DrawRoundRect(Graphics g, Pen pen, float x, float y, float width, float height, float radius)
{
GraphicsPath path = new GraphicsPath();
// 添加左上角弧线
path.AddArc(x, y, radius * 2, radius * 2, 180, 90);
// 添加上边线
path.AddLine(x + radius, y, x + width - radius, y);
// 添加右上角弧线
path.AddArc(x + width - radius * 2, y, radius * 2, radius * 2, 270, 90);
// 添加右边线
path.AddLine(x + width, y + radius, x + width, y + height - radius);
// 添加右下角弧线
path.AddArc(x + width - radius * 2, y + height - radius * 2, radius * 2, radius * 2, 0, 90);
// 添加下边线
path.AddLine(x + width - radius, y + height, x + radius, y + height);
// 添加左下角弧线
path.AddArc(x, y + height - radius * 2, radius * 2, radius * 2, 90, 90);
// 关闭路径
path.CloseFigure();
// 绘制路径
g.DrawPath(pen, path);
}
```
在 `Form` 的 `Paint` 事件中调用该方法即可:
```csharp
private void Form1_Paint(object sender, PaintEventArgs e)
{
Pen pen = new Pen(Color.Red, 2);
DrawRoundRect(e.Graphics, pen, 50, 50, 200, 100, 20);
pen.Dispose();
}
```
这将绘制一个红色边框、圆角半径为20的矩形。
阅读全文
相关推荐
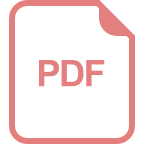

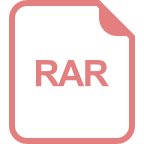
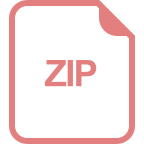
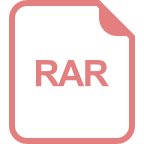

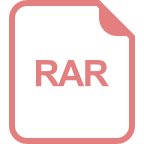
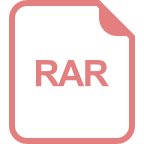
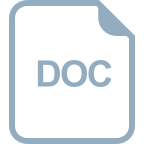
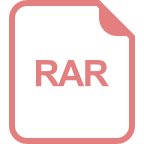
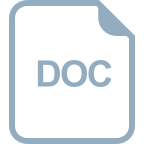
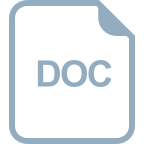
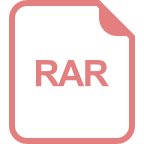
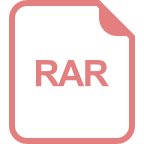
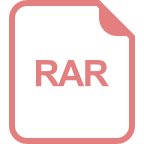
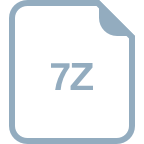
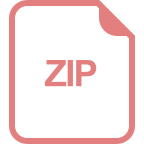