#include<iostream> using namespace std; #include<string.h> class name { public: name(char* pn); ~name(); protected: char* pname; int size; }; name::name(char* pn) { cout << " Constructing " << pn << endl; pname = new char[strlen(pn) + 1]; if (pname != 0) strcpy(pname, pn); //字符串放到pname内存 size = strlen(pn); } name :: ~name() { cout << " Destructing " << pname << endl; delete[]pname; size = 0; } int main(int argc, char* argv[]) { name Obj1("NoName"); name Obj2 = Obj1; return 0; }
时间: 2024-04-07 15:32:08 浏览: 115
这是一个 C++ 的程序,定义了一个类 name,其中包含了一个构造函数和一个析构函数。构造函数的作用是在对象被创建时初始化对象的成员变量,析构函数的作用是在对象被销毁时释放内存。在主函数中,定义了两个对象 Obj1 和 Obj2,其中 Obj2 是通过拷贝构造函数创建的。这个程序的作用主要是演示了对象的构造和析构过程。
相关问题
#include<iostream> using namespace std; class Car { char* pName; int id; int m; public: Car(string name) { } }; 怎么将string name转换为char*pName
您可以使用`c_str()`函数将`std::string`类型转换为`const char*`类型,然后再将其复制到`char*`类型的变量中。下面是修改后的代码:
```
#include <iostream>
using namespace std;
class Car {
char* pName;
int id;
int m;
public:
Car(string name) {
pName = new char[name.length() + 1];
strcpy(pName, name.c_str());
id = 0;
m = 0;
}
~Car() {
delete[] pName;
}
};
int main() {
Car car("My car");
return 0;
}
```
在构造函数中,我们使用`new char[name.length() + 1]`为`pName`分配内存,该内存可以容纳字符串及其终止符。然后我们使用`strcpy()`函数将`name.c_str()`复制到`pName`中。最后,在析构函数中,我们使用`delete[]`释放内存。
定义一个描述学生基本情况的类,数据成员包括:年龄、姓名、性别;成员函数包括:输入和输出各个数据成员的值。 分别给出如下的3个文件: ①含类定义的头文件student.h, //student.h class Student { public: void display( ); private: int num; char name[20]; char sex; }; ②包含成员函数定义的源文件student.cpp //student.cpp #include <iostream> using namespace std; #include "student.h" void Student::display( ) { cout<<"num: "<<num<<endl; cout<<"name: "<<name<<endl; cout<<"sex: "<<sex<<endl; } ③包含主函数的源文件main.cpp。 为了组成一个完整的源程序,应当有包括主函数的源文件: //main.cpp #include <iostream> using namespace std; #include "student.h" int main( ) {Student stud; stud.display(); return 0; } 请完善该程序,在类中增加一个对数据成员赋初值的成员函数set_value。修改补充代码并上机运行
/student.h
```c++
class Student {
public:
void display();
void set_value(int n, char *nam, char s);
private:
int num;
char name[20];
char sex;
};
```
/student.cpp
```c++
#include <iostream>
#include "student.h"
using namespace std;
void Student::display() {
cout << "num: " << num << endl;
cout << "name: " << name << endl;
cout << "sex: " << sex << endl;
}
void Student::set_value(int n, char *nam, char s) {
num = n;
strcpy(name, nam);
sex = s;
}
```
/main.cpp
```c++
#include <iostream>
#include "student.h"
using namespace std;
int main() {
Student stud;
stud.set_value(1001, "Tom", 'M');
stud.display();
return 0;
}
```
阅读全文
相关推荐
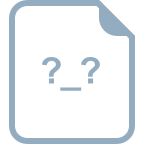
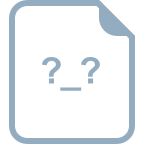


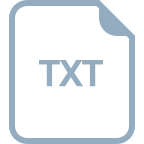
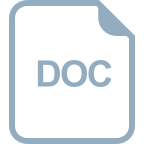
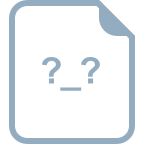
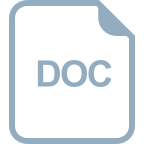
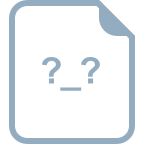
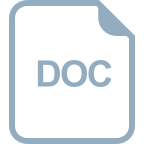
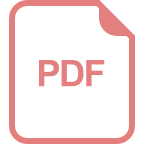
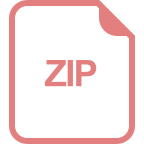
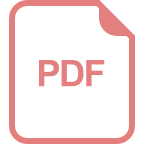
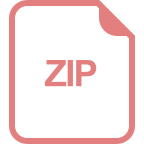