Java实现布尔模型检索
时间: 2023-11-13 22:08:02 浏览: 36
布尔模型检索是一种基于逻辑运算的信息检索方法,其中文档和查询都被表示为布尔表达式,并使用布尔运算符(AND、OR、NOT)进行操作。
以下是一个简单的 Java 代码示例,用于实现布尔模型检索:
```java
import java.util.*;
public class BooleanRetrieval {
public static void main(String[] args) {
// 创建文档集合
Map<String, Set<Integer>> index = new HashMap<>();
index.put("apple", new HashSet<>(Arrays.asList(2, 4, 5)));
index.put("banana", new HashSet<>(Arrays.asList(1, 3, 5)));
index.put("orange", new HashSet<>(Arrays.asList(2, 4)));
index.put("pear", new HashSet<>(Arrays.asList(1, 5)));
// 查询
String query = "apple AND orange OR NOT banana";
Set<Integer> result = search(query, index);
// 打印结果
System.out.println(result);
}
public static Set<Integer> search(String query, Map<String, Set<Integer>> index) {
Stack<Set<Integer>> stack = new Stack<>();
for (String token : query.split("\\s+")) {
if (token.equals("AND")) {
stack.push(intersect(stack.pop(), stack.pop()));
} else if (token.equals("OR")) {
stack.push(union(stack.pop(), stack.pop()));
} else if (token.equals("NOT")) {
stack.push(not(stack.pop(), index.keySet()));
} else {
stack.push(index.getOrDefault(token, Collections.emptySet()));
}
}
if (stack.size() != 1) {
throw new IllegalArgumentException("Invalid query: " + query);
}
return stack.pop();
}
public static Set<Integer> intersect(Set<Integer> left, Set<Integer> right) {
Set<Integer> result = new HashSet<>(left);
result.retainAll(right);
return result;
}
public static Set<Integer> union(Set<Integer> left, Set<Integer> right) {
Set<Integer> result = new HashSet<>(left);
result.addAll(right);
return result;
}
public static Set<Integer> not(Set<Integer> set, Set<String> universe) {
Set<Integer> result = new HashSet<>();
for (int i = 1; i <= universe.size(); i++) {
if (!set.contains(i)) {
result.add(i);
}
}
return result;
}
}
```
在此示例中,我们首先创建了一个包含文档的索引,然后执行一个查询。查询使用布尔运算符“AND”、“OR”和“NOT”来组合单词,并调用 search() 函数来获取结果。
search() 函数使用一个栈来处理查询。它遍历查询中的每个单词,并根据操作符(AND、OR 或 NOT)执行相应的操作。对于每个单词,它检查索引并将相关文档的 ID 添加到栈中。
最后,search() 函数从栈中弹出最终结果并返回。
intersect()、union() 和 not() 函数用于实现集合操作。
请注意,此示例仅适用于小型数据集。对于大型数据集,您可能需要使用更高效的数据结构和算法来实现布尔模型检索。
相关推荐
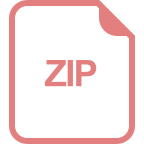
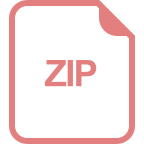
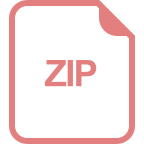














