用Python写一个希尔密码加密解密
时间: 2024-10-23 14:05:51 浏览: 32
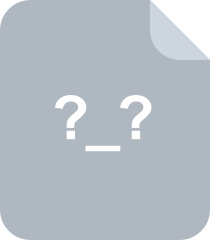
希尔密码解密脚本Python
希尔密码(Hill Cipher),也称矩阵密码,是一种基于线性代数的替换式加密方法。在Python中实现希尔密码,首先需要定义矩阵操作以及处理字母表转换。
以下是一个简单的希尔密码加密解密示例:
```python
import numpy as np
def shift_matrix(matrix, key):
return (matrix @ key) % 26
def encrypt(text, key_matrix):
text = list(text.upper()) # 转换成大写字母并转换成字符列表
n = len(key_matrix)
encrypted_text = []
for i in range(0, len(text), n):
chunk = text[i:i+n]
matrix_chunk = [ord(char) - ord('A') for char in chunk] # 字符转数字(A=0, Z=25)
encrypted_chunk = shift_matrix(np.array(matrix_chunk).reshape(n, n), key_matrix)
encrypted_text.extend([chr((char + 26) % 26 + ord('A')) for char in encrypted_chunk.flatten()])
return ''.join(encrypted_text)
def decrypt(encrypted_text, key_matrix):
return encrypt(encrypted_text, np.linalg.inv(key_matrix))
# 示例
key = [[5, 8], [17, 3]]
plaintext = "Hello, World!"
ciphertext = encrypt(plaintext, key)
print(f"Encrypted: {ciphertext}")
decrypted_text = decrypt(ciphertext, key)
print(f"Decrypted: {decrypted_text}")
```
在这个例子中,我们使用一个2x2的矩阵作为密钥,并将文本分为块进行加密。注意,为了保持希尔密码的稳定性和安全性,实际应用中会使用更大、更复杂的矩阵,同时密钥的选择也很关键。
阅读全文
相关推荐
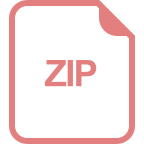
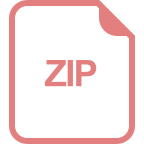
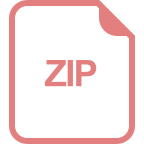
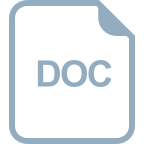






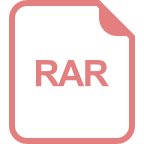
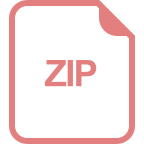
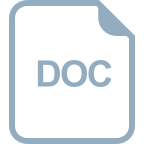




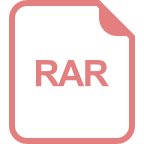
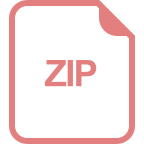