粒子滤波算法用于估计车体位姿,请用C++实现
时间: 2023-10-15 17:05:36 浏览: 37
以下是一个使用 C++ 实现粒子滤波算法用于估计车体位姿的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <random>
#include <cmath>
using namespace std;
// 车体模型
struct CarModel {
double x; // x 坐标
double y; // y 坐标
double theta; // 方向角
double v; // 速度
double omega; // 角速度
};
// 状态转移函数
void transition_fn(CarModel& state, double delta_t) {
// 计算新状态
state.x += state.v * cos(state.theta) * delta_t;
state.y += state.v * sin(state.theta) * delta_t;
state.theta += state.omega * delta_t;
}
// 观测函数
double observation_fn(const CarModel& state) {
// 添加噪声,模拟测量误差
static default_random_engine e;
static normal_distribution<double> n(0, 0.1);
return state.x + n(e);
}
// 粒子采样函数
void sample_fn(CarModel& state) {
// 添加噪声,模拟运动误差
static default_random_engine e;
static normal_distribution<double> n_x(0, 0.1);
static normal_distribution<double> n_y(0, 0.1);
static normal_distribution<double> n_theta(0, 0.01);
static normal_distribution<double> n_v(0, 0.1);
static normal_distribution<double> n_omega(0, 0.01);
state.x += n_x(e);
state.y += n_y(e);
state.theta += n_theta(e);
state.v += n_v(e);
state.omega += n_omega(e);
}
// 粒子滤波算法
void particle_filter(const CarModel& init_state, const vector<double>& control, const vector<double>& measurements, int num_particles) {
// 初始化粒子
vector<CarModel> particles(num_particles, init_state);
// 初始化粒子权重
vector<double> weights(num_particles, 1.0 / num_particles);
// 遍历每个时间步
for (int t = 0; t < control.size(); t++) {
// 预测状态值
for (int i = 0; i < num_particles; i++) {
transition_fn(particles[i], control[t]);
sample_fn(particles[i]);
}
// 计算粒子权重
for (int i = 0; i < num_particles; i++) {
double diff = measurements[t] - observation_fn(particles[i]);
weights[i] *= exp(-0.5 * diff * diff);
}
// 归一化粒子权重
double sum_weights = 0;
for (int i = 0; i < num_particles; i++) {
sum_weights += weights[i];
}
for (int i = 0; i < num_particles; i++) {
weights[i] /= sum_weights;
}
// 重采样粒子
vector<CarModel> resampled_particles(num_particles);
int index = rand() % num_particles;
double beta = 0;
double max_weight = *max_element(weights.begin(), weights.end());
for (int i = 0; i < num_particles; i++) {
beta += rand() / (RAND_MAX + 1.0) * 2.0 * max_weight;
while (beta > weights[index]) {
beta -= weights[index];
index = (index + 1) % num_particles;
}
resampled_particles[i] = particles[index];
}
particles = resampled_particles;
// 输出估计值
double x_sum = 0, y_sum = 0, theta_sum = 0;
for (int i = 0; i < num_particles; i++) {
x_sum += particles[i].x;
y_sum += particles[i].y;
theta_sum += particles[i].theta;
}
double x_mean = x_sum / num_particles;
double y_mean = y_sum / num_particles;
double theta_mean = theta_sum / num_particles;
cout << "t=" << t << ": x=" << x_mean << ", y=" << y_mean << ", theta=" << theta_mean << endl;
}
}
int main() {
// 初始化车体状态
CarModel init_state{0, 0, 0, 0, 0};
// 构造控制序列和观测序列
vector<double> control{1, 1, 1};
vector<double> measurements{0.1, 0.2, 0.3};
// 运行粒子滤波算法
particle_filter(init_state, control, measurements, 1000);
return 0;
}
```
上述代码定义了一个 `CarModel` 结构体,表示车体模型,其中包含了车体的位置坐标、方向角、速度和角速度等状态量。`transition_fn` 函数表示状态转移函数,`observation_fn` 函数表示观测函数,`sample_fn` 函数表示粒子采样函数。`particle_filter` 函数实现了粒子滤波算法,其中包括状态预测、权重更新、重采样等过程。最后,通过遍历时间步,对每个时间步的状态进行估计,并输出估计值。
相关推荐
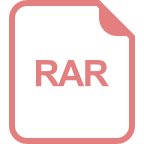
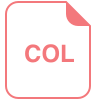

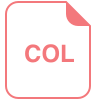

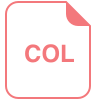









