sqlserver 根据实体类public class ReportMenu { /报表id/ private Integer id; /报表分组/ private String reportGroup; /报表名称/ private String reportName; /报表路径/ private String reportUrl; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getReportGroup() { return reportGroup; } public void setReportGroup(String reportGroup) { this.reportGroup = reportGroup; } public String getReportName() { return reportName; } public void setReportName(String reportName) { this.reportName = reportName; } public String getReportUrl() { return reportUrl; } public void setReportUrl(String reportUrl) { this.reportUrl = reportUrl; } }构建建表sql,要求驼峰命名改下划线,并添加字段备注
时间: 2024-01-09 07:04:22 浏览: 140
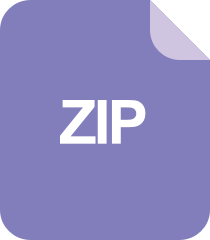
BeetlSQL的基本调用
根据上述实体类,建表 SQL 如下所示:
```
CREATE TABLE report_menu (
id INT NOT NULL COMMENT '报表id',
report_group VARCHAR(50) NOT NULL COMMENT '报表分组',
report_name VARCHAR(50) NOT NULL COMMENT '报表名称',
report_url VARCHAR(100) NOT NULL COMMENT '报表路径',
PRIMARY KEY (id)
);
```
在建表 SQL 中,将实体类中的驼峰命名改为了下划线命名,并添加了每个字段的注释说明。注意,主键的约束也需要在 SQL 中体现,这里使用了 `PRIMARY KEY` 关键字来声明 id 字段为主键。
阅读全文
相关推荐
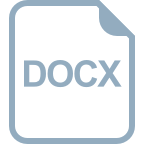
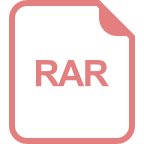

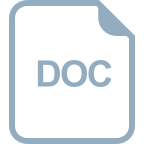
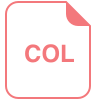













