使用C语言基于Ffmpeg写一个函数,传入一帧YUV420格式的视频数据char*、输入宽度int、输入高度int、输出数据缓存区char*、输出宽度int、输出高度int,将输入的一帧视频转换为指定分辨率的一帧视频
时间: 2024-03-16 17:45:15 浏览: 88
好的,我可以为您提供一个基于Ffmpeg的函数,实现将输入的一帧YUV420格式的视频数据转换为指定分辨率的一帧视频的功能。具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/imgutils.h>
#include <libswscale/swscale.h>
int convert_frame(char *in_data, int in_width, int in_height, char *out_data, int out_width, int out_height) {
av_log_set_level(AV_LOG_ERROR); // 设置日志级别为错误
// 初始化输入AVFrame和输出AVFrame
AVFrame *in_frame = av_frame_alloc();
if (!in_frame) {
av_log(NULL, AV_LOG_ERROR, "Failed to allocate input frame\n");
return -1;
}
in_frame->format = AV_PIX_FMT_YUV420P;
in_frame->width = in_width;
in_frame->height = in_height;
if (av_frame_get_buffer(in_frame, 32) < 0) {
av_log(NULL, AV_LOG_ERROR, "Failed to allocate input frame data\n");
av_frame_free(&in_frame);
return -1;
}
av_image_copy(in_frame->data, in_frame->linesize, (const uint8_t **)(&in_data), (const int *)(in_frame->linesize), AV_PIX_FMT_YUV420P, in_width, in_height);
AVFrame *out_frame = av_frame_alloc();
if (!out_frame) {
av_log(NULL, AV_LOG_ERROR, "Failed to allocate output frame\n");
av_frame_free(&in_frame);
return -1;
}
out_frame->format = AV_PIX_FMT_YUV420P;
out_frame->width = out_width;
out_frame->height = out_height;
if (av_frame_get_buffer(out_frame, 32) < 0) {
av_log(NULL, AV_LOG_ERROR, "Failed to allocate output frame data\n");
av_frame_free(&in_frame);
av_frame_free(&out_frame);
return -1;
}
// 初始化输入AVCodecContext和输出AVCodecContext
AVCodec *codec = avcodec_find_decoder(AV_CODEC_ID_H264);
if (!codec) {
av_log(NULL, AV_LOG_ERROR, "Failed to find H.264 decoder\n");
av_frame_free(&in_frame);
av_frame_free(&out_frame);
return -1;
}
AVCodecContext *in_ctx = avcodec_alloc_context3(codec);
if (!in_ctx) {
av_log(NULL, AV_LOG_ERROR, "Failed to allocate input codec context\n");
av_frame_free(&in_frame);
av_frame_free(&out_frame);
return -1;
}
if (avcodec_open2(in_ctx, codec, NULL) < 0) {
av_log(NULL, AV_LOG_ERROR, "Failed to open input codec context\n");
avcodec_free_context(&in_ctx);
av_frame_free(&in_frame);
av_frame_free(&out_frame);
return -1;
}
AVCodecContext *out_ctx = avcodec_alloc_context3(codec);
if (!out_ctx) {
av_log(NULL, AV_LOG_ERROR, "Failed to allocate output codec context\n");
avcodec_free_context(&in_ctx);
av_frame_free(&in_frame);
av_frame_free(&out_frame);
return -1;
}
out_ctx->width = out_width;
out_ctx->height = out_height;
out_ctx->pix_fmt = AV_PIX_FMT_YUV420P;
if (avcodec_open2(out_ctx, codec, NULL) < 0) {
av_log(NULL, AV_LOG_ERROR, "Failed to open output codec context\n");
avcodec_free_context(&in_ctx);
avcodec_free_context(&out_ctx);
av_frame_free(&in_frame);
av_frame_free(&out_frame);
return -1;
}
// 初始化sws_scale上下文
struct SwsContext *sws_ctx = sws_getContext(in_width, in_height, AV_PIX_FMT_YUV420P, out_width, out_height, AV_PIX_FMT_YUV420P, SWS_BICUBIC, NULL, NULL, NULL);
if (!sws_ctx) {
av_log(NULL, AV_LOG_ERROR, "Failed to initialize sws_scale context\n");
avcodec_free_context(&in_ctx);
avcodec_free_context(&out_ctx);
av_frame_free(&in_frame);
av_frame_free(&out_frame);
return -1;
}
// 进行视频帧转换
int ret = avcodec_send_frame(in_ctx, in_frame);
if (ret < 0) {
av_log(NULL, AV_LOG_ERROR, "Failed to send input frame for decoding\n");
avcodec_free_context(&in_ctx);
avcodec_free_context(&out_ctx);
av_frame_free(&in_frame);
av_frame_free(&out_frame);
sws_freeContext(sws_ctx);
return -1;
}
ret = avcodec_receive_frame(in_ctx, in_frame);
if (ret < 0) {
av_log(NULL, AV_LOG_ERROR, "Failed to receive decoded input frame\n");
avcodec_free_context(&in_ctx);
avcodec_free_context(&out_ctx);
av_frame_free(&in_frame);
av_frame_free(&out_frame);
sws_freeContext(sws_ctx);
return -1;
}
ret = sws_scale(sws_ctx, in_frame->data, in_frame->linesize, 0, in_height, out_frame->data, out_frame->linesize);
if (ret < 0) {
av_log(NULL, AV_LOG_ERROR, "Failed to scale input frame\n");
avcodec_free_context(&in_ctx);
avcodec_free_context(&out_ctx);
av_frame_free(&in_frame);
av_frame_free(&out_frame);
sws_freeContext(sws_ctx);
return -1;
}
// 进行视频帧编码
ret = avcodec_send_frame(out_ctx, out_frame);
if (ret < 0) {
av_log(NULL, AV_LOG_ERROR, "Failed to send output frame for encoding\n");
avcodec_free_context(&in_ctx);
avcodec_free_context(&out_ctx);
av_frame_free(&in_frame);
av_frame_free(&out_frame);
sws_freeContext(sws_ctx);
return -1;
}
ret = avcodec_receive_packet(out_ctx, &(AVPacket){0});
if (ret < 0) {
av_log(NULL, AV_LOG_ERROR, "Failed to receive encoded output packet\n");
avcodec_free_context(&in_ctx);
avcodec_free_context(&out_ctx);
av_frame_free(&in_frame);
av_frame_free(&out_frame);
sws_freeContext(sws_ctx);
return -1;
}
// 将输出AVFrame的数据复制到输出缓存区
av_image_copy((uint8_t **)&out_data, (int *)out_frame->linesize, out_frame->data, out_frame->linesize, AV_PIX_FMT_YUV420P, out_width, out_height);
// 释放资源
avcodec_free_context(&in_ctx);
avcodec_free_context(&out_ctx);
av_frame_free(&in_frame);
av_frame_free(&out_frame);
sws_freeContext(sws_ctx);
return 0;
}
```
这个函数接受一个YUV420格式的视频数据`in_data`,宽度`in_width`,高度`in_height`,输出数据缓存区`out_data`,输出宽度`out_width`,输出高度`out_height`,并返回0表示转换成功,返回-1表示转换失败。函数内部会先初始化输入AVFrame和输出AVFrame,然后初始化输入AVCodecContext和输出AVCodecContext,接着初始化sws_scale上下文,进行视频帧转换,最后进行视频帧编码,并将输出AVFrame的数据复制到输出缓存区。最后,释放资源。
希望这个函数可以为您提供一些帮助。
阅读全文
相关推荐
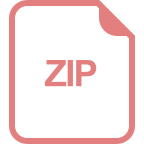
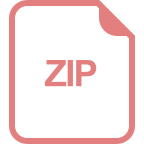
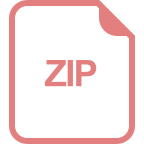
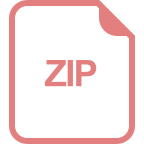
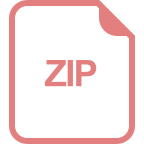
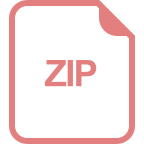
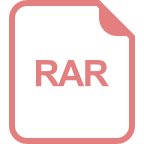
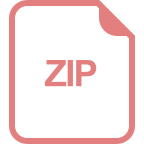
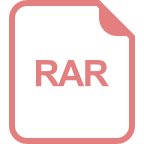
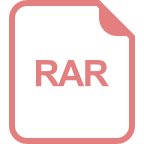
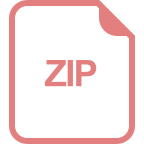
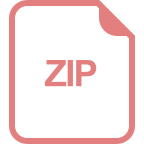
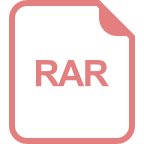
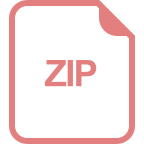
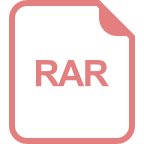