C/c++获取网络使用率
时间: 2023-08-03 08:05:25 浏览: 242
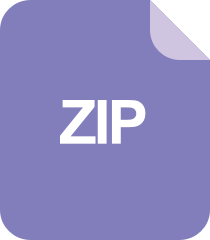
VC++ 测量网络时延与利用率

要获取网络使用率,可以通过以下步骤使用C/C++编写程序:
1. 使用系统函数获取网络流量信息,例如在Windows平台上,可以使用GetIfTable和GetIfEntry函数获取网络接口信息和流量统计数据。
2. 计算网络使用率,可以使用流量统计数据计算网络使用率,通常使用以下公式:使用率 = (接收字节数 + 发送字节数) / 带宽 * 时间间隔。
3. 可以使用定时器或循环方式来周期性地获取和计算网络使用率。
以下是一个简单的C++示例代码,可以获取并打印出当前网络使用率:
```c++
#include <iostream>
#include <windows.h>
#include <iphlpapi.h>
#pragma comment(lib, "iphlpapi.lib")
int main()
{
MIB_IFTABLE* ifTable = nullptr;
DWORD ifTableSize = 0;
// 获取网络接口信息
GetIfTable(ifTable, &ifTableSize, FALSE);
if (ifTableSize == 0)
{
std::cerr << "Failed to get interface table!" << std::endl;
return -1;
}
ifTable = (MIB_IFTABLE*)new char[ifTableSize];
if (GetIfTable(ifTable, &ifTableSize, FALSE) != NO_ERROR)
{
std::cerr << "Failed to get interface table!" << std::endl;
delete[] ifTable;
return -1;
}
// 计算网络使用率
ULONG totalBytes = 0;
ULONG totalBandwidth = 0;
for (int i = 0; i < ifTable->dwNumEntries; ++i)
{
MIB_IFROW& ifRow = ifTable->table[i];
if (ifRow.dwType == MIB_IF_TYPE_ETHERNET)
{
totalBytes += ifRow.dwInOctets + ifRow.dwOutOctets;
totalBandwidth += ifRow.dwSpeed;
}
}
static ULONG lastTotalBytes = 0;
static ULONG lastTime = GetTickCount();
ULONG currentTime = GetTickCount();
ULONG elapsedTime = (currentTime - lastTime) / 1000;
ULONG currentBytes = totalBytes - lastTotalBytes;
lastTotalBytes = totalBytes;
lastTime = currentTime;
float usage = (float)currentBytes / totalBandwidth * 8 / elapsedTime;
std::cout << "Network usage: " << usage << " Mbps" << std::endl;
delete[] ifTable;
return 0;
}
```
需要注意的是,代码中仅仅统计了Ethernet接口的流量信息,并且只计算了单向流量,实际应用中可能需要根据具体需求进行修改。
阅读全文
相关推荐

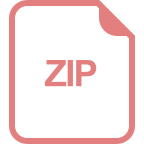
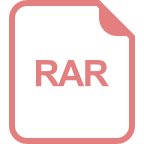
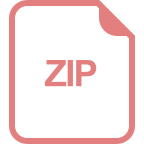
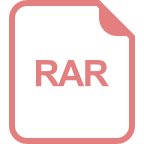
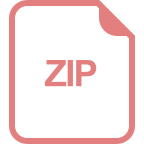
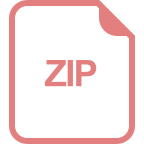
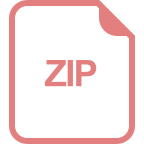
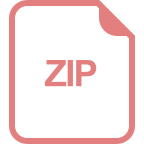
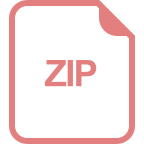
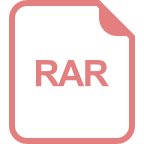
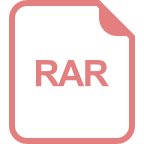
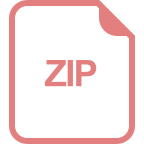
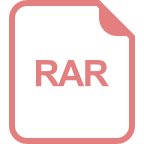
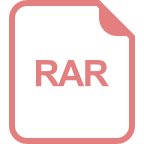
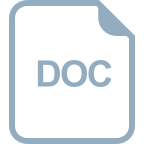