用Pygame做一个2048
时间: 2023-03-20 16:03:29 浏览: 95
好的,下面是一个简单的2048游戏的Pygame实现:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 游戏面板大小
BOARD_SIZE = (400, 400)
# 方格大小
CELL_SIZE = 100
# 游戏面板背景颜色
BACKGROUND_COLOR = (187, 173, 160)
# 方格颜色
CELL_COLORS = {
2: (238, 228, 218),
4: (237, 224, 200),
8: (242, 177, 121),
16: (245, 149, 99),
32: (246, 124, 95),
64: (246, 94, 59),
128: (237, 207, 114),
256: (237, 204, 97),
512: (237, 200, 80),
1024: (237, 197, 63),
2048: (237, 194, 46),
}
# 字体颜色
FONT_COLOR = (255, 255, 255)
# 字体大小
FONT_SIZE = 40
# 创建游戏面板
screen = pygame.display.set_mode(BOARD_SIZE)
# 设置游戏标题
pygame.display.set_caption('2048')
# 创建字体对象
font = pygame.font.SysFont('Arial', FONT_SIZE)
# 生成方格矩阵
board = [[0 for x in range(4)] for y in range(4)]
def draw_board():
"""
绘制游戏面板和方格
"""
screen.fill(BACKGROUND_COLOR)
for y in range(4):
for x in range(4):
value = board[y][x]
color = CELL_COLORS.get(value, (205, 193, 180))
rect = pygame.Rect(x * CELL_SIZE, y * CELL_SIZE, CELL_SIZE, CELL_SIZE)
pygame.draw.rect(screen, color, rect)
if value:
text = font.render(str(value), True, FONT_COLOR)
text_rect = text.get_rect(center=rect.center)
screen.blit(text, text_rect)
def add_random():
"""
在空位置随机添加数字2或4
"""
x = random.randint(0, 3)
y = random.randint(0, 3)
while board[y][x] != 0:
x = random.randint(0, 3)
y = random.randint(0, 3)
board[y][x] = random.choice([2, 4])
def merge(row):
"""
将一行中的方格向左合并
"""
i = 0
while i < len(row) - 1:
if row[i] == row[i + 1]:
row[i] *= 2
row[i + 1] = 0
i += 1
return row
def move_left():
"""
将所有方格向左移动并合并
"""
global board
new_board = []
for row in board:
new_row = [x for x in row if x != 0]
new_row += [0] * (4 - len(new_row))
new_row = merge(new_row)
new_board.append(new_row)
if board != new_board:
board = new_board
阅读全文
相关推荐
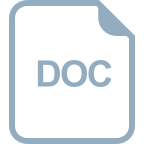
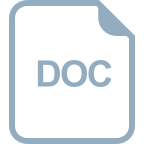
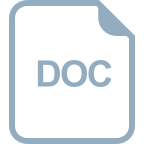

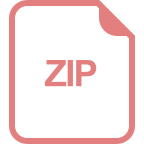
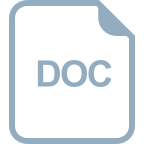





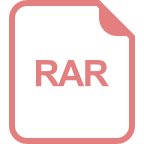
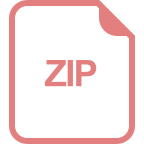
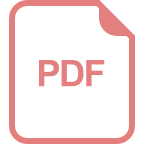
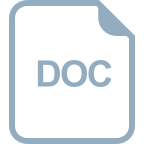


