Vgg加FPN和ResNet加FPN后 在分类前融合
时间: 2024-05-31 13:10:07 浏览: 18
这两种方法的主要目的是增强图像分类的准确性。其中,Vgg加FPN和ResNet加FPN属于特征金字塔网络(Feature Pyramid Network,简称FPN)的两种变体,它们在不同的卷积神经网络上应用了FPN方法。
Vgg加FPN和ResNet加FPN后,在分类前融合的方法是将两个网络的特征图进行融合,然后再进行分类。这种方法可以充分利用不同层次的特征信息,从而提高图像分类的准确性。
具体来说,该方法会首先将图像输入到Vgg或ResNet网络中,得到不同层次的特征图。然后,将这些特征图输入到FPN网络中,进行特征融合。最后,将融合后的特征图输入到分类器中进行分类。
需要注意的是,Vgg加FPN和ResNet加FPN后,在分类前融合的方法需要训练大量的参数,因此需要大量的数据和计算资源。同时,该方法的计算复杂度较高,需要较长的训练时间。
相关问题
Vgg加FPN和ResNet加FPN后 在分类前融合 代码
以下是使用PyTorch实现VGG和ResNet与FPN的融合的代码:
``` python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torchvision.models import vgg16, resnet50
class VGG_FPN(nn.Module):
def __init__(self, num_classes=1000):
super(VGG_FPN, self).__init__()
vgg = vgg16(pretrained=True)
self.features = vgg.features
self.fpn_layers = nn.Sequential(
nn.Conv2d(512, 256, kernel_size=1, stride=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 512, kernel_size=1, stride=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Conv2d(512, 1024, kernel_size=1, stride=1),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
nn.Conv2d(1024, 1024, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True)
)
self.avgpool = nn.AdaptiveAvgPool2d((7, 7))
self.classifier = nn.Sequential(
nn.Dropout(),
nn.Linear(1024 * 7 * 7, 4096),
nn.ReLU(inplace=True),
nn.Dropout(),
nn.Linear(4096, 4096),
nn.ReLU(inplace=True),
nn.Linear(4096, num_classes)
)
def forward(self, x):
x = self.features(x)
c3, c4, c5 = x.size()
p5 = self.fpn_layers(x)
p4 = F.upsample(p5, size=(c4), mode="nearest") + self.fpn_layers(x)
p3 = F.upsample(p4, size=(c3), mode="nearest") + self.fpn_layers(x)
x = F.adaptive_avg_pool2d(p3, output_size=(1, 1))
x = x.view(x.size(0), -1)
x = self.classifier(x)
return x
class ResNet_FPN(nn.Module):
def __init__(self, num_classes=1000):
super(ResNet_FPN, self).__init__()
resnet = resnet50(pretrained=True)
self.conv1 = resnet.conv1
self.bn1 = resnet.bn1
self.relu = resnet.relu
self.maxpool = resnet.maxpool
self.layer1 = resnet.layer1
self.layer2 = resnet.layer2
self.layer3 = resnet.layer3
self.layer4 = resnet.layer4
self.fpn_layers = nn.Sequential(
nn.Conv2d(2048, 256, kernel_size=1, stride=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 512, kernel_size=1, stride=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Conv2d(512, 1024, kernel_size=1, stride=1),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
nn.Conv2d(1024, 1024, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True)
)
self.avgpool = nn.AdaptiveAvgPool2d((7, 7))
self.fc = nn.Linear(1024 * 7 * 7, num_classes)
def forward(self, x):
x = self.conv1(x)
x = self.bn1(x)
x = self.relu(x)
x = self.maxpool(x)
c2 = self.layer1(x)
c3 = self.layer2(c2)
c4 = self.layer3(c3)
c5 = self.layer4(c4)
p5 = self.fpn_layers(c5)
p4 = F.upsample(p5, size=(c4.size()[2], c4.size()[3]), mode="nearest") + self.fpn_layers(c4)
p3 = F.upsample(p4, size=(c3.size()[2], c3.size()[3]), mode="nearest") + self.fpn_layers(c3)
x = F.adaptive_avg_pool2d(p3, output_size=(1, 1))
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
```
以上代码实现了VGG和ResNet与FPN的融合,其中VGG_FPN和ResNet_FPN分别代表融合后的模型。模型分为特征提取层和分类层两部分,特征提取层通过VGG或ResNet提取特征,然后再使用FPN将多层特征融合。分类层则将融合后的特征作为输入,输出分类结果。注意,这里使用了nn.Sequential()将多个卷积层组成了一个整体。
VGG16和ResNet101_FPN哪个速度快
在图像识别领域,VGG16通常比ResNet101_FPN快。这是因为VGG16具有较少的层和参数,因此在推理时需要较少的计算量。相比之下,ResNet101_FPN具有更深的架构和更多的参数,因此需要更多的计算。但是,在其他任务,如目标检测和语义分割中,ResNet101_FPN通常比VGG16表现更好,并且速度也相对较快。
相关推荐
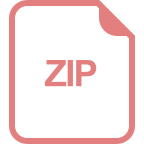
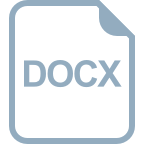
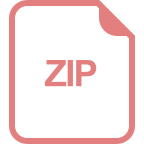












