用c++编写一个过载函数,能够针对不同类型的引用传递变量
时间: 2024-02-05 17:12:57 浏览: 22
可以使用 C++ 中的模板来实现过载函数,针对不同类型的引用传递变量。以下是一个简单的示例代码:
```c++
#include <iostream>
template<typename T>
void printRef(T& val) {
std::cout << "LValue reference: " << val << std::endl;
}
template<typename T>
void printRef(T&& val) {
std::cout << "RValue reference: " << val << std::endl;
}
int main() {
int x = 10;
int& ref = x;
const int& cref = 20;
printRef(x); // LValue reference: 10
printRef(ref); // LValue reference: 10
printRef(cref); // LValue reference: 20
printRef(30); // RValue reference: 30
printRef(std::move(x)); // RValue reference: 10
return 0;
}
```
在上面的代码中,我们定义了两个模板函数 `printRef`,一个接受左值引用,另一个接受右值引用。当我们调用 `printRef` 函数时,编译器会根据传递的参数类型自动选择合适的函数进行调用。由于模板函数可以推断出参数类型,因此我们可以在不同类型的引用传递变量时使用该函数。
相关推荐
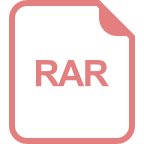
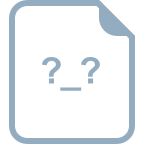
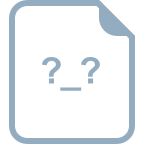
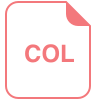
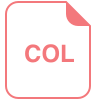

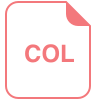
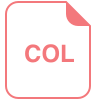









