Collectors.toMap Function.identity()
时间: 2023-12-01 14:43:41 浏览: 45
`Collectors.toMap(Function.identity())`是一个用于将流中的元素收集到一个Map中的方法,其中`Function.identity()`表示使用元素本身作为Map的key。例如,以下代码将一个字符串列表转换为一个Map,其中字符串本身是key,字符串长度是value:
```java
List<String> list = Arrays.asList("apple", "banana", "orange");
Map<String, Integer> map = list.stream().collect(Collectors.toMap(Function.identity(), String::length));
```
这将生成一个Map,其中键是字符串本身,值是字符串的长度。在这个例子中,Map的内容将是:
```
{apple=5, banana=6, orange=6}
```
相关问题
Collectors.toMap value泛型
Collectors.toMap方法是Java 8中提供的用于将流(Stream)中的元素收集到Map中的方法。它有多个重载的方法,其中一个重要的参数是valueMapper,用于指定如何将流中的元素映射为Map中的值。
在Collectors.toMap方法中,valueMapper参数是一个Function类型的参数,用于将流中的元素映射为Map中的值。该函数接受一个元素作为输入,并返回一个值作为输出。在使用Collectors.toMap方法时,我们可以通过lambda表达式或方法引用来指定valueMapper参数。
在指定valueMapper时,我们可以使用泛型来定义返回值的类型。例如,如果我们有一个流中的元素类型为String,我们可以使用泛型来指定valueMapper返回的值类型为Integer。示例代码如下:
```
List<String> strings = Arrays.asList("apple", "banana", "cherry");
Map<String, Integer> map = strings.stream()
.collect(Collectors.toMap(Function.identity(), String::length));
```
在上述示例中,我们将字符串流中的每个元素作为Map的键,并将其长度作为值。这里的valueMapper参数使用了方法引用String::length,它将字符串映射为其长度(Integer类型)。
Collectors.toMap
### 回答1:
Collectors.toMap 是 Java 8 中的一个静态方法,用于将一个 Stream 中的元素转换为一个 Map 对象。它接受三个参数:
1. keyMapper:一个 Function 接口的实现,用于将 Stream 中的元素转换为 Map 的 key。
2. valueMapper:一个 Function 接口的实现,用于将 Stream 中的元素转换为 Map 的 value。
3. mergeFunction:一个 BinaryOperator 接口的实现,用于处理当 key 值重复时的冲突。
示例代码:
```java
List<String> list = Arrays.asList("apple", "banana", "orange");
Map<String, Integer> map = list.stream().collect(Collectors.toMap(Function.identity(), String::length));
```
这段代码将 List 中的每个元素作为 key,将每个元素的长度作为 value,最终得到一个 Map 对象。其中,Function.identity() 返回一个接受任何参数并返回该参数的函数,即将元素本身作为 key。String::length 是一个方法引用,表示将元素的长度作为 value。由于这个例子中每个元素都不相同,所以不需要 mergeFunction。如果有重复的 key,可以使用 mergeFunction 处理冲突。
### 回答2:
Collectors.toMap是Java 8中的一个静态方法,用于将流(Stream)中的元素转化为一个Map数据结构。它有两个重载的方法:
1. toMap(Function<? super T, ? extends K> keyMapper, Function<? super T, ? extends U> valueMapper)
这个方法接收两个函数参数,一个用于将流元素映射为Map的键,另一个用于将流元素映射为Map的值。它会返回一个包含流元素作为键和值的Map。
2. toMap(Function<? super T, ? extends K> keyMapper, Function<? super T, ? extends U> valueMapper, BinaryOperator<U> mergeFunction)
这个方法还接收一个合并函数参数,用于解决流中存在重复键时的冲突。它会返回一个包含合并后的键值对的Map。
使用Collectors.toMap需要注意以下几点:
- 流中的元素不能为null,否则会抛出NullPointerException。
- 流中的元素的键不能重复,否则会抛出IllegalStateException,解决方法是使用第二个重载方法,并提供一个合并函数。
- 返回的Map类型取决于流的类型,如果流是有序的,返回的Map将保留原始顺序,否则返回的Map顺序无法保证。
下面是一个使用Collectors.toMap的简单示例:
```
List<String> fruits = Arrays.asList("apple", "banana", "orange");
Map<String, Integer> fruitLengthMap = fruits.stream()
.collect(Collectors.toMap(Function.identity(), String::length));
System.out.println(fruitLengthMap);
```
输出结果:
```
{apple=5, orange=6, banana=6}
```
以上代码将水果列表转化为以水果名称作为键,长度作为值的Map。
### 回答3:
Collectors.toMap是Java 8中Stream API提供的一个收集器,它用于将一个流(Stream)中的元素根据指定的键和值的提取函数,转换为一个Map对象。
使用Collectors.toMap方法,我们可以根据流中的元素创建一个Map对象。该方法接受两个参数:一个是键的提取函数,用于从流中的每个元素中提取键;另一个是值的提取函数,用于从流中的每个元素中提取值。
例如,假设我们有一个商品类Product,其中包含商品名称和价格属性。我们可以使用Collectors.toMap方法将商品的名称作为键,价格作为值,将商品列表转换为一个以商品名称为键、价格为值的Map对象。
下面是一个使用Collectors.toMap的示例代码:
```java
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List<Product> products = Arrays.asList(
new Product("手机", 1000),
new Product("电视", 2000),
new Product("电脑", 3000)
);
Map<String, Integer> productMap = products.stream()
.collect(Collectors.toMap(Product::getName, Product::getPrice));
System.out.println(productMap);
}
}
class Product {
private String name;
private int price;
public Product(String name, int price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public int getPrice() {
return price;
}
}
```
输出结果为:
```
{手机=1000, 电视=2000, 电脑=3000}
```
在上面的示例中,我们使用Collectors.toMap方法将商品列表转换为了一个以商品名称为键、价格为值的Map对象。
相关推荐
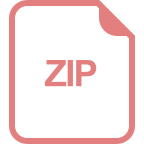
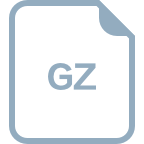












