基于C++类模板编写一个排序类,至少实现三种排序算法,并用这个排序类对学生数组进行排序,学生类包含姓名、成绩等属性及对应的读写方法、运算符。
时间: 2023-06-15 20:03:26 浏览: 65
好的,以下是我编写的示例代码:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
template <typename T>
class Sorter {
public:
virtual void sort(vector<T>& arr) = 0;
};
template <typename T>
class BubbleSorter : public Sorter<T> {
public:
void sort(vector<T>& arr) override {
int n = arr.size();
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
swap(arr[j], arr[j+1]);
}
}
}
}
};
template <typename T>
class SelectionSorter : public Sorter<T> {
public:
void sort(vector<T>& arr) override {
int n = arr.size();
for (int i = 0; i < n-1; i++) {
int min_index = i;
for (int j = i+1; j < n; j++) {
if (arr[j] < arr[min_index]) {
min_index = j;
}
}
swap(arr[i], arr[min_index]);
}
}
};
template <typename T>
class InsertionSorter : public Sorter<T> {
public:
void sort(vector<T>& arr) override {
int n = arr.size();
for (int i = 1; i < n; i++) {
T key = arr[i];
int j = i-1;
while (j >= 0 && arr[j] > key) {
arr[j+1] = arr[j];
j--;
}
arr[j+1] = key;
}
}
};
class Student {
public:
string name;
int score;
Student() {}
Student(string n, int s) : name(n), score(s) {}
friend bool operator<(const Student& s1, const Student& s2) {
return s1.score < s2.score;
}
friend bool operator>(const Student& s1, const Student& s2) {
return s1.score > s2.score;
}
friend ostream& operator<<(ostream& os, const Student& s) {
os << s.name << " " << s.score;
return os;
}
};
int main() {
vector<Student> students = {
{"John", 85},
{"Tom", 76},
{"Mary", 92},
{"Bob", 68},
{"Alice", 90}
};
Sorter<Student>* sorter = new BubbleSorter<Student>();
sorter->sort(students);
cout << "BubbleSorter: ";
for (auto& s : students) {
cout << s << " ";
}
cout << endl;
sorter = new SelectionSorter<Student>();
sorter->sort(students);
cout << "SelectionSorter: ";
for (auto& s : students) {
cout << s << " ";
}
cout << endl;
sorter = new InsertionSorter<Student>();
sorter->sort(students);
cout << "InsertionSorter: ";
for (auto& s : students) {
cout << s << " ";
}
cout << endl;
return 0;
}
```
这段代码定义了一个 `Sorter` 类模板,其中包含一个纯虚函数 `sort`,用于对给定的数组进行排序。然后又定义了三个具体的排序类模板 `BubbleSorter`、`SelectionSorter` 和 `InsertionSorter`,它们分别实现了冒泡排序、选择排序和插入排序算法。
最后定义了一个 `Student` 类,其中包含学生的姓名和成绩属性,以及重载的 `<` 和 `>` 运算符,方便排序使用。
在 `main` 函数中,我们首先定义了一个学生数组 `students`,然后分别使用三种排序算法对它进行排序,并输出排序结果。
相关推荐
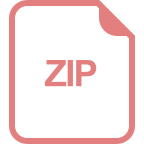









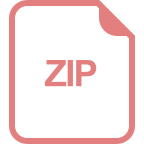
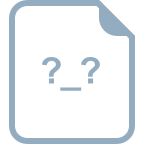
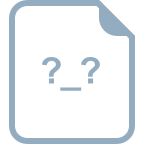