% Component initialization methods (Access = private) % Create UIFigure and components function createComponents(app) % Get the file path for locating images pathToMLAPP = fileparts(mfilename('fullpath')); % Create RangeFindingUIFigure and hide until all components are created app.RangeFindingUIFigure = uifigure('Visible', 'off'); app.RangeFindingUIFigure.Position = [100 100 542 362]; app.RangeFindingUIFigure.Name = 'Range Finding'; app.RangeFindingUIFigure.Icon = fullfile(pathToMLAPP, '1251215.png'); % Create UIAxes app.UIAxes = uiaxes(app.RangeFindingUIFigure); title(app.UIAxes, '输入') zlabel(app.UIAxes, 'Z') app.UIAxes.XTick = []; app.UIAxes.XTickLabel = ''; app.UIAxes.YTick = []; app.UIAxes.ZTick = []; app.UIAxes.Position = [36 117 230 185]; % Create UIAxes_2 app.UIAxes_2 = uiaxes(app.RangeFindingUIFigure); title(app.UIAxes_2, '输出') zlabel(app.UIAxes_2, 'Z') app.UIAxes_2.XTick = []; app.UIAxes_2.XTickLabel = ''; app.UIAxes_2.YTick = []; app.UIAxes_2.ZTick = []; app.UIAxes_2.Position = [287 117 230 185]; % Create Button app.Button = uibutton(app.RangeFindingUIFigure, 'push'); app.Button.ButtonPushedFcn = createCallbackFcn(app, @ButtonPushed, true); app.Button.Position = [118 47 63 23]; app.Button.Text = '选择图像'; % Create Button_2 app.Button_2 = uibutton(app.RangeFindingUIFigure, 'push'); app.Button_2.ButtonPushedFcn = createCallbackFcn(app, @Button_2Pushed, true); app.Button_2.Position = [375 47 52 23]; app.Button_2.Text = '识别'; % Create Label app.Label = uilabel(app.RangeFindingUIFigure); app.Label.HorizontalAlignment = 'right'; app.Label.Position = [208 320 69 22]; app.Label.Text = '距离(cm)'; % Create cmEditField app.cmEditField = uieditfield(app.RangeFindingUIFigure, 'numeric'); app.cmEditField.Position = [292 320 44 22]; % Show the figure after all components are created app.RangeFindingUIFigure.Visible = 'on'; end end
时间: 2023-06-20 15:07:13 浏览: 226
这段代码是用 MATLAB App Designer 创建图形用户界面(GUI)的组件。其中包含了两个 UIAxes,分别用于显示输入图像和输出图像,两个 Button 分别用于选择图像和进行识别,一个 Label 用于显示距离,一个 cmEditField 用于输入距离。这些组件的位置、大小、文字和回调函数都在代码中设置。
阅读全文
相关推荐






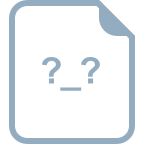
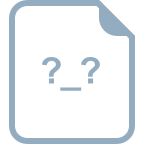









